C# 多线程ping并写入文件
时间: 2023-10-30 17:04:52 浏览: 56
以下是使用 C# 多线程实现 ping 并将结果写入文件的示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.IO;
using System.Net;
using System.Net.NetworkInformation;
using System.Threading;
class Program
{
static void Main(string[] args)
{
List<string> hostList = new List<string> { "www.google.com", "www.yahoo.com", "www.bing.com" };
string fileName = "ping_results.txt";
using (StreamWriter writer = new StreamWriter(fileName))
{
foreach (string host in hostList)
{
ThreadPool.QueueUserWorkItem(new WaitCallback(PingHost), new object[] { host, writer });
}
Console.WriteLine("Ping in progress...");
Console.ReadLine();
}
}
static void PingHost(object data)
{
string host = (string)((object[])data)[0];
StreamWriter writer = (StreamWriter)((object[])data)[1];
Ping pingSender = new Ping();
PingOptions options = new PingOptions();
options.DontFragment = true;
string dataString = "abcdefghijklmnopqrstuvwxyz";
byte[] buffer = Encoding.ASCII.GetBytes(dataString);
int timeout = 120;
PingReply reply = pingSender.Send(host, timeout, buffer, options);
if (reply.Status == IPStatus.Success)
{
Console.WriteLine("{0} is reachable ({1} ms)", host, reply.RoundtripTime);
writer.WriteLine("{0} is reachable ({1} ms)", host, reply.RoundtripTime);
}
else
{
Console.WriteLine("{0} is not reachable ({1})", host, reply.Status.ToString());
writer.WriteLine("{0} is not reachable ({1})", host, reply.Status.ToString());
}
}
}
```
在此示例中,我们首先定义一个包含主机列表和输出文件名的变量。然后,我们使用 StreamWriter 打开输出文件,并使用 ThreadPool.QueueUserWorkItem 方法将每个主机的 Ping 任务添加到线程池中以进行并发执行。最后,我们等待用户按下 Enter 键以继续执行程序。
在 PingHost 方法中,我们创建一个 Ping 对象并使用指定的主机名、超时时间和数据缓冲区发送 Ping 请求。如果 Ping 请求成功,则记录主机的可达性和往返时间。否则,记录主机的不可达原因。
请注意,我们使用 StreamWriter 的线程安全实现,以确保多个线程可以同时写入文件,而不会产生竞争条件。
相关推荐
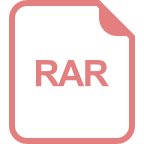














