Python 对高光谱图像进行混合像元分解的详细代码
时间: 2024-10-14 22:16:31 浏览: 147
在Python中,对高光谱图像进行混合像元分解通常使用线性代数库如NumPy和Scikit-image。一个常见的混合像元分解方法是独立成分分析(Independent Component Analysis, ICA)。以下是一个简化的例子,展示了如何使用FastICA函数来进行高光谱图像的混合像元分解:
```python
import numpy as np
from sklearn.decomposition import FastICA
# 假设你的高光谱图像数据存储在数组hs_data中
# 这里假设它是二维数组,每个像素是一维向量
hs_data = ... # 你需要的实际高光谱数据
# 将数据转换成适合FastICA的形状(样本数 x 特征数)
n_samples, n_bands = hs_data.shape
hs_data_reshaped = np.reshape(hs_data, (n_samples, -1))
ica = FastICA(n_components=n_bands)
decomposed_data = ica.fit_transform(hs_data_reshaped)
# decomposed_data now contains the independent components
# 每行代表一个独立的像元成分
# 可能还会对结果进行后续处理,比如归一化或可视化
...
相关问题
python混合像元分解实例代码
Python中混合像素分解(Mixture Modeling)通常用于图像分割或数据分析,比如高光谱数据处理。Pandas和scikit-learn库可以辅助我们进行这样的操作,这里我将提供一个基于高斯混合模型(Gaussian Mixture Model,GMM)的简单例子:
```python
# 导入所需库
import numpy as np
from sklearn.mixture import GaussianMixture
import matplotlib.pyplot as plt
# 假设我们有二维高光谱数据
data = np.random.rand(1000, 2) # 创建随机数据点
# 定义GMM模型,假设我们想找出3种混合成分
n_components = 3
gmm = GaussianMixture(n_components=n_components)
# 训练模型
gmm.fit(data)
# 预测每个数据点属于哪一种混合分布
labels = gmm.predict(data)
# 绘制原始数据和分割结果
plt.scatter(data[:, 0], data[:, 1], c=labels)
plt.title('Mixed Pixel Decomposition using GMM')
plt.xlabel('Feature 1')
plt.ylabel('Feature 2')
# 显示图
plt.show()
混合像元分解FCLS的python实现代码
混合像元分解(FCLS, Feature Linear Combinations of Subspaces)是一种非负矩阵分解技术,它通常用于信号处理、图像分析等领域,尤其是在高光谱遥感数据降维和特征提取中。Python中可以利用Scikit-learn库等工具来实现FCLS算法。
以下是一个简单的FCLS的Python实现示例,假设我们有一个高光谱图像数组`spectral_data`:
```python
from sklearn.decomposition import NMF
import numpy as np
# 假设spectral_data是一个二维数组,每一行代表一个像素,每列对应一个波段
spectral_data = ... # 你需要替换为你实际的数据
# 定义FCLS函数
def fcls(spectral_data, n_components):
nmf = NMF(n_components=n_components, init='nndsvd', non_negative=True)
# 使用NMF对数据进行分解
feature_combinations = nmf.fit_transform(spectral_data)
return feature_combinations
# 调用FCLS函数并获取结果
feature_vectors = fcls(spectral_data, n_components=5)
阅读全文
相关推荐
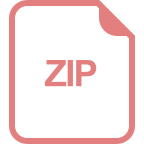
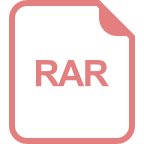
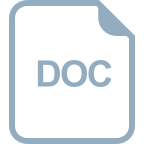
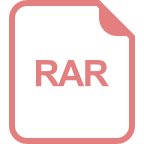
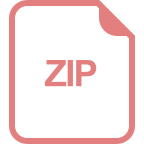
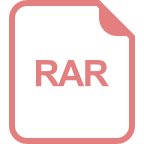
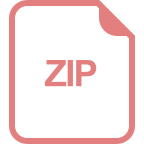
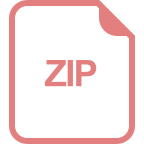
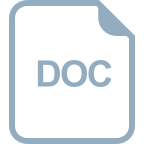
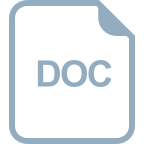
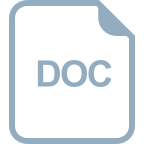
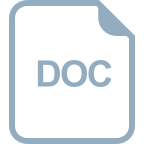
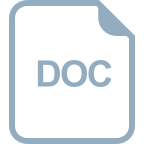
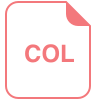
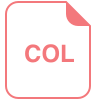
