normalized_beat_2d[i] = np.zeros((len(normalized_beat[i]),), dtype=object) normalized_beat_2d[i] = np.zeros((len(normalized_beat[i]), 2)) normalized_beat_2d[i][:, 0] = normalized_beat[i] normalized_beat_2d[i][:, 1] = (i / 255) mtf = MarkovTransitionField() image[i] = mtf.transform(normalized_beat_2d[i])之后怎么保存马尔可夫变化生成的图像
时间: 2023-12-10 17:41:54 浏览: 78
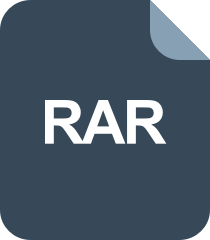
MATLAB.rar_Normalized_cut segmentation_matlab normalized c_norma
你可以使用Python中的PIL库或OpenCV库将生成的图像保存为图像文件,比如PNG、JPEG等格式。下面是使用PIL库保存图像的示例代码:
```python
from PIL import Image
# 假设生成的图像为image[i]
# 将图像转换为PIL Image对象
pil_image = Image.fromarray(image[i])
# 保存图像到文件
pil_image.save('output.png')
```
你也可以使用OpenCV库保存图像,示例代码如下:
```python
import cv2
# 假设生成的图像为image[i]
# 保存图像到文件
cv2.imwrite('output.png', image[i])
```
注意,保存图像时需要指定文件名和文件格式。如果文件名后缀和实际保存的图像格式不一致,可能会导致无法正常打开图像文件。
阅读全文
相关推荐
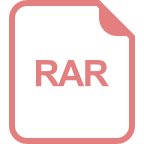
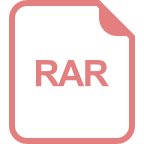



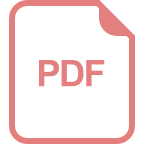
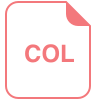
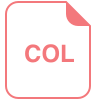




