Vector(int n=SPARE_MAX):: n(max) ①//转换构造函数,参数初始化列表 这个错了请改正
时间: 2024-02-05 17:12:45 浏览: 133
这个代码中的错误有两个:
1. `SPARE_MAX` 未定义,需要先进行定义或者包含定义该常量的头文件。
2. 在转换构造函数中,应该调用基础类的默认构造函数来初始化对象,而不是使用参数初始化列表来初始化。
因此,正确的代码应该是:
```
Vector(int n = 0) : elem{new T[n]}, sz{n}, space{n > 4 ? n : DEFAULT_SIZE} {}
```
其中,`DEFAULT_SIZE` 是一个常量,表示默认的数组大小。
相关问题
优化这段代码 import heapq import numpy as np def MinTimeSlot(containerList, req, CONTAINER_SPARE): seq1 = 0 spareContainerList = [] for container in containerList: if container.appId == req.appId and container.state == CONTAINER_SPARE: seq1 += 1 heapq.heappush(spareContainerList, (-1 * container.killedTime, seq1, container)) return spareContainerList
这段代码的优化方案需要结合具体使用场景和数据规模来考虑。以下是几个可能的优化方案:
1. 使用列表推导式替代循环,可以增加代码的可读性和执行效率:
```
seq1 = 0
spareContainerList = [(-1 * container.killedTime, i, container) for i, container in enumerate(containerList) if container.appId == req.appId and container.state == CONTAINER_SPARE]
heapq.heapify(spareContainerList)
return spareContainerList
```
2. 如果列表中的元素数量很大,可以考虑使用生成器表达式和堆排序算法来节省内存和提高执行速度:
```
def MinTimeSlot(containerList, req, CONTAINER_SPARE):
spareContainerList = ((-1 * container.killedTime, i, container) for i, container in enumerate(containerList) if container.appId == req.appId and container.state == CONTAINER_SPARE)
return heapq.nsmallest(len(spareContainerList), spareContainerList)
```
3. 如果该函数需要频繁调用,可以将已经筛选出来的容器列表缓存起来,避免重复计算:
```
class ContainerCache:
def __init__(self, containerList):
self.containerDict = {}
for container in containerList:
if container.appId not in self.containerDict:
self.containerDict[container.appId] = []
if container.state == CONTAINER_SPARE:
self.containerDict[container.appId].append(container)
def get_spare_containers(self, appId):
return self.containerDict.get(appId, [])
containerCache = ContainerCache(containerList)
def MinTimeSlot(containerList, req, CONTAINER_SPARE, containerCache):
seq1 = 0
spareContainerList = []
for container in containerCache.get_spare_containers(req.appId):
seq1 += 1
heapq.heappush(spareContainerList, (-1 * container.killedTime, seq1, container))
return spareContainerList
```
解释 server: tomcat: uri-encoding: UTF-8 threads: max: 200 min-spare: 30 connection-timeout: 5000ms port: 8080 servlet: context-path: /Csdn-api
在上述配置中,"server" 是一个部分,它用于配置服务器的相关设置。其中,"tomcat" 是一个服务器容器,用于托管和处理 Web 应用程序。在这个配置中,"uri-encoding" 设置为 UTF-8,表示请求的 URI 编码采用 UTF-8 编码格式。
"threads" 部分用于配置服务器处理请求的线程池。"max" 表示线程池中的最大线程数为 200,"min-spare" 表示最小空闲线程数为 30。这些配置可根据应用程序的需求进行调整,以提高服务器的性能和并发处理能力。
"connection-timeout" 用于设置连接超时时间,这里设置为 5000 毫秒(5 秒)。如果连接在此时间内没有建立成功,则会超时。
"port" 配置了服务器监听的端口号,这里设置为 8080。
"servlet" 部分用于配置 Servlet 相关的设置。"context-path" 表示应用程序的上下文路径,这里设置为 "/Csdn-api"。当访问应用程序时,需要通过此路径进行访问。
总的来说,上述配置是为了定制化服务器的行为和性能,以适应特定的应用程序需求。
阅读全文
相关推荐
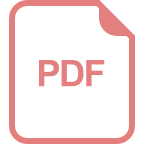
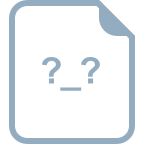
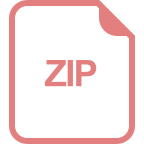
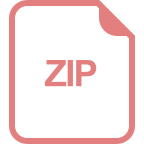
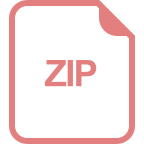
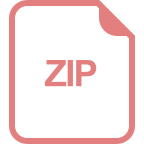
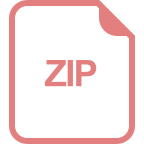
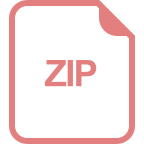
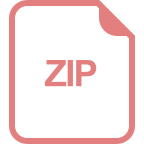
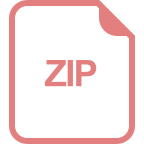
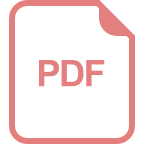
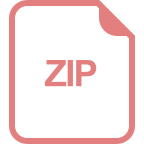
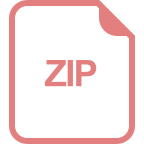


