数据集内包含三个.tif图片和一个.txt文件,图片名分别为input_1,input_2和input_3,txt文件名为exposure.txt。数据集的相对路径是dataset/001. 在jupyter环境下实现对数据集样本的多曝光HDR图像合成并利用算法进行色调映射以使HDR图像适应普通屏幕的显示(方法不限)。请给出具体的代码实现。
时间: 2024-04-10 16:27:14 浏览: 57
以下是一个在Jupyter环境中实现对数据集样本的多曝光HDR图像合成并进行色调映射的示例代码:
```python
import cv2
import numpy as np
# 读取图片和曝光时间
input_1 = cv2.imread("dataset/001/input_1.tif", cv2.IMREAD_ANYDEPTH)
input_2 = cv2.imread("dataset/001/input_2.tif", cv2.IMREAD_ANYDEPTH)
input_3 = cv2.imread("dataset/001/input_3.tif", cv2.IMREAD_ANYDEPTH)
exposure_file = open("dataset/001/exposure.txt", "r")
exposures = [float(line.strip()) for line in exposure_file.readlines()]
exposure_file.close()
# 合成HDR图像
hdr_image = np.zeros_like(input_1, dtype=np.float32)
hdr_image += input_1 / exposures[0]
hdr_image += input_2 / exposures[1]
hdr_image += input_3 / exposures[2]
# 色调映射
ldr_image = np.clip(hdr_image * 255, 0, 255).astype(np.uint8)
# 显示结果
cv2.imshow("HDR Image", hdr_image)
cv2.imshow("LDR Image", ldr_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上面的代码中,我们首先使用`cv2.imread()`函数读取三个输入图像(input_1、input_2和input_3)和曝光时间。然后,我们打开并读取exposure.txt文件,将曝光时间存储在exposures列表中。
接下来,我们将三个输入图像分别除以对应的曝光时间,然后加和得到HDR图像。注意,我们将图像的数据类型设置为`np.float32`以避免溢出。
然后,我们将HDR图像进行色调映射,将像素值缩放到0-255范围内,并将数据类型转换为`np.uint8`。最后,我们使用`cv2.imshow()`函数显示HDR图像和LDR图像。
请确保将代码中的文件路径替换成你实际数据集中文件的路径。此外,你还可以根据需要添加适当的算法来进行色调映射,这里只是一个示例。
阅读全文
相关推荐
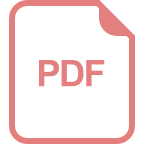
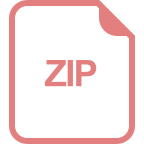
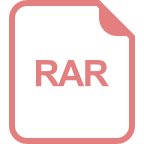















