用C++语言写一个完整代码 在主函数中,可以创建一个指向 Book 类的指针数组,然后动态地创建 Book 类的对象和 EBook 类的对象,将这些对象存储到指针数组中。然后,可以通过循环来调用每个对象的 print() 函数,实现多态输出。 另外,可以编写一个静态函数 menu(),用于输出操作菜单,让用户选择要进行的操作,例如添加图书、删除图书等。
时间: 2023-02-13 16:48:21 浏览: 100
#include <iostream>
#include <string>
#include <vector>
// 定义基类 Book
class Book {
public:
Book(std::string book_name, std::string book_author, int book_page_count)
: name(book_name),
author(book_author),
page_count(book_page_count) {}
virtual void Print() const {
std::cout << "Book name: " << name << std::endl;
std::cout << "Author: " << author << std::endl;
std::cout << "Page count: " << page_count << std::endl;
}
private:
std::string name;
std::string author;
int page_count;
};
// 定义派生类 EBook
class EBook : public Book {
public:
EBook(std::string book_name, std::string book_author, int book_page_count,
std::string book_format, int book_size)
: Book(book_name, book_author, book_page_count),
format(book_format),
size(book_size) {}
void Print() const override {
Book::Print();
std::cout << "Format: " << format << std::endl;
std::cout << "Size: " << size << std::endl;
}
private:
std::string format;
int size;
};
// 定义静态函数 menu
static void menu() {
std::cout << "Please choose an option:" << std::endl;
std::cout << "1. Add a book" << std::endl;
std::cout << "2. Add an ebook" << std::endl;
std::cout << "3. Delete a book" << std::endl;
std::cout << "4. Print all books" << std::endl;
std::cout << "5. Quit" << std::endl;
}
int main() {
std::vector<Book*> books; // 创建指向 Book 类的指针数组
while (true) {
menu(); // 输出操作菜单
int option;
std::cin >> option;
if (option == 1) {
std::cout << "Please enter the name of the book: ";
std::string name;
std::cin >> name;
std::cout << "Please enter the author of the book: ";
std::string author;
std::cin >> author;
std::cout << "Please enter the page count of the book: ";
int page_count;
std::cin >> page_count;
books.push_back(new Book(name, author, page_count)); // 动态地创建 Book 类的对象
} else if (option == 2) {
std
阅读全文
相关推荐
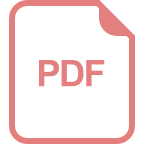
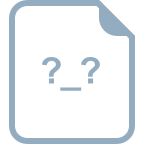
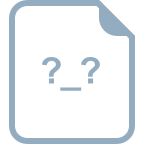








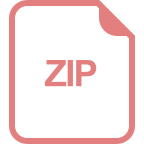
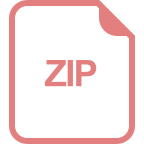
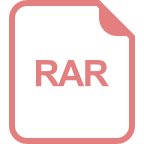
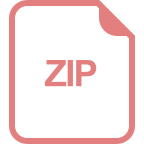
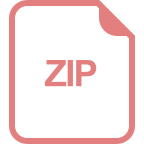
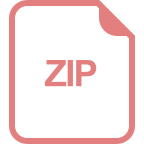