定义图的邻接表存储结构,并编写图的初始化、建立图、输出图、广度优先遍历、计算并输出图中每个顶点的度等基本操作实现函数。同时在主函数中调用这些函数进行验证的实现源代码
时间: 2024-02-02 08:05:09 浏览: 23
定义图的邻接表存储结构:
```c++
#define MAX_VERTEX_NUM 100 // 最大顶点数
typedef struct ArcNode {
int adjvex; // 邻接点在数组中的位置下标
struct ArcNode *nextarc; // 指向下一个邻接点的指针
} ArcNode;
typedef struct VNode {
char data; // 顶点信息
ArcNode *firstarc; // 指向第一个邻接点的指针
} VNode, AdjList[MAX_VERTEX_NUM];
typedef struct {
AdjList vertices; // 图的顶点数组
int vexnum, arcnum; // 图的当前顶点数和弧数
} ALGraph;
```
初始化一个空图:
```c++
void InitGraph(ALGraph &G) {
G.vexnum = G.arcnum = 0;
for (int i = 0; i < MAX_VERTEX_NUM; i++) {
G.vertices[i].data = '\0';
G.vertices[i].firstarc = NULL;
}
}
```
根据输入构建图:
```c++
void CreateGraph(ALGraph &G) {
printf("请输入顶点数和边数:\n");
scanf("%d%d", &G.vexnum, &G.arcnum);
getchar(); // 吃掉回车符
printf("请输入顶点信息:\n");
for (int i = 0; i < G.vexnum; i++) {
scanf("%c", &G.vertices[i].data);
G.vertices[i].firstarc = NULL;
}
getchar(); // 吃掉回车符
printf("请输入边的信息:\n");
for (int i = 0; i < G.arcnum; i++) {
char v1, v2;
int pos1, pos2;
printf("请输入第%d条边的两个顶点信息:\n", i + 1);
scanf("%c%c", &v1, &v2);
getchar(); // 吃掉回车符
pos1 = LocateVex(G, v1);
pos2 = LocateVex(G, v2);
ArcNode *p = (ArcNode*)malloc(sizeof(ArcNode));
p->adjvex = pos2;
p->nextarc = G.vertices[pos1].firstarc;
G.vertices[pos1].firstarc = p;
p = (ArcNode*)malloc(sizeof(ArcNode));
p->adjvex = pos1;
p->nextarc = G.vertices[pos2].firstarc;
G.vertices[pos2].firstarc = p;
}
}
```
输出图的邻接表:
```c++
void PrintGraph(ALGraph G) {
printf("图的邻接表:\n");
for (int i = 0; i < G.vexnum; i++) {
printf("%c -> ", G.vertices[i].data);
ArcNode *p = G.vertices[i].firstarc;
while (p) {
printf("%c -> ", G.vertices[p->adjvex].data);
p = p->nextarc;
}
printf("NULL\n");
}
}
```
广度优先遍历:
```c++
void BFS(ALGraph G, int v, bool visited[]) {
queue<int> q;
printf("%c ", G.vertices[v].data);
visited[v] = true;
q.push(v);
while (!q.empty()) {
int u = q.front();
q.pop();
ArcNode *p = G.vertices[u].firstarc;
while (p) {
int w = p->adjvex;
if (!visited[w]) {
printf("%c ", G.vertices[w].data);
visited[w] = true;
q.push(w);
}
p = p->nextarc;
}
}
}
```
计算并输出图中每个顶点的度:
```c++
void PrintDegree(ALGraph G) {
printf("每个顶点的度:\n");
for (int i = 0; i < G.vexnum; i++) {
int degree = 0;
ArcNode *p = G.vertices[i].firstarc;
while (p) {
degree++;
p = p->nextarc;
}
printf("%c: %d\n", G.vertices[i].data, degree);
}
}
```
主函数:
```c++
int main() {
ALGraph G;
InitGraph(G);
CreateGraph(G);
PrintGraph(G);
printf("广度优先遍历结果:\n");
bool visited[MAX_VERTEX_NUM] = { false };
for (int i = 0; i < G.vexnum; i++) {
if (!visited[i]) {
BFS(G, i, visited);
}
}
printf("\n");
PrintDegree(G);
return 0;
}
```
相关推荐
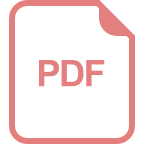
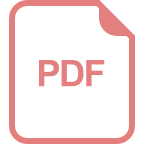
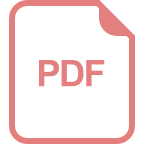














