定义图的邻接表存储结构,并编写创建图、输出图、广度优先遍历、计算并输出图中每个顶点的度等基本操作实现函数。同时在主函数中调用这些函数进行验证
时间: 2024-02-12 07:09:51 浏览: 72
以下是图的邻接表存储结构定义和基本操作实现函数:
```C++
#include <iostream>
#include <queue>
using namespace std;
// 定义边结构体
struct Edge {
int dest; // 目标节点
int weight; // 权重
Edge* next; // 链表下一个节点
Edge(int dest, int weight) : dest(dest), weight(weight), next(NULL) {}
};
// 定义图结构体
struct Graph {
int V; // 节点数量
Edge** adjList; // 邻接表数组
// 图构造函数
Graph(int V) : V(V) {
adjList = new Edge*[V];
for (int i = 0; i < V; i++) {
adjList[i] = NULL;
}
}
// 添加边
void addEdge(int src, int dest, int weight) {
// 创建新边
Edge* newEdge = new Edge(dest, weight);
// 把新边插入到邻接表中
newEdge->next = adjList[src];
adjList[src] = newEdge;
}
// 输出图
void printGraph() {
for (int i = 0; i < V; i++) {
cout << "节点 " << i << " 的邻接表为:";
Edge* cur = adjList[i];
while (cur) {
cout << cur->dest << "(" << cur->weight << ") ";
cur = cur->next;
}
cout << endl;
}
}
// 计算每个顶点的度
void calculateDegrees() {
for (int i = 0; i < V; i++) {
int degree = 0;
Edge* cur = adjList[i];
while (cur) {
degree++;
cur = cur->next;
}
cout << "节点 " << i << " 的度为:" << degree << endl;
}
}
// 广度优先遍历
void BFS(int start) {
// 初始化 visited 数组
bool visited[V];
for (int i = 0; i < V; i++) {
visited[i] = false;
}
// 创建队列
queue<int> q;
// 将起点入队
q.push(start);
visited[start] = true;
// 开始遍历
while (!q.empty()) {
int cur = q.front();
q.pop();
cout << cur << " ";
// 遍历邻接表中的所有节点
Edge* curEdge = adjList[cur];
while (curEdge) {
int next = curEdge->dest;
if (!visited[next]) {
// 如果该节点未被访问过,则将其入队
visited[next] = true;
q.push(next);
}
curEdge = curEdge->next;
}
}
cout << endl;
}
};
// 创建图
Graph createGraph() {
int V, E;
cout << "请输入节点数量和边数量:";
cin >> V >> E;
Graph g(V);
cout << "请依次输入每条边的起点、终点、权重:" << endl;
for (int i = 0; i < E; i++) {
int src, dest, weight;
cin >> src >> dest >> weight;
g.addEdge(src, dest, weight);
}
return g;
}
int main() {
Graph g = createGraph();
cout << "图的邻接表如下:" << endl;
g.printGraph();
cout << "图的广度优先遍历结果为:";
g.BFS(0);
cout << "图中每个顶点的度为:" << endl;
g.calculateDegrees();
return 0;
}
```
在主函数中,我们首先调用 `createGraph()` 创建图,然后调用 `printGraph()` 输出图的邻接表,接着调用 `BFS()` 对图进行广度优先遍历,最后调用 `calculateDegrees()` 计算并输出每个顶点的度。
阅读全文
相关推荐
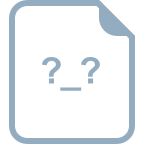














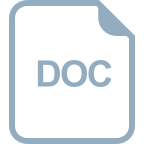
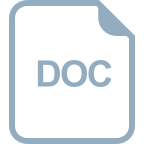