用c语言实现,题目如下:内容:构建图的邻接矩阵、邻接表存储结构,实现图的深度优先搜索遍历、广度优先搜索遍历。 要求: (1)创建图时,输入图中的顶点以及图中的边(或弧)。 (2)输出图的深度优先遍历序列和广度优先遍历序列。
时间: 2024-02-13 13:02:39 浏览: 90
好的,我会尽力回答这个问题。
首先,我们需要定义一个图的结构体,包括顶点数、边数以及邻接矩阵和邻接表两种数据结构:
```c
#define MAX_VERTEX_NUM 100 // 最大顶点数
// 边的结构体
typedef struct {
int start; // 起点
int end; // 终点
int weight; // 权值(如果是无权图,则为1)
} Edge;
// 图的结构体
typedef struct {
int vertex_num; // 顶点数
int edge_num; // 边数
int adj_matrix[MAX_VERTEX_NUM][MAX_VERTEX_NUM]; // 邻接矩阵
struct {
int vertex; // 顶点编号
struct EdgeNode* first_edge; // 指向第一条依附该顶点的边的指针
} adj_list[MAX_VERTEX_NUM]; // 邻接表
} Graph;
```
接下来,我们需要实现图的创建函数,可以通过用户输入来构建图的邻接矩阵和邻接表:
```c
void createGraph(Graph* graph) {
printf("请输入图的顶点数和边数(用空格隔开):");
scanf("%d %d", &graph->vertex_num, &graph->edge_num);
// 初始化邻接矩阵
for (int i = 0; i < graph->vertex_num; i++) {
for (int j = 0; j < graph->vertex_num; j++) {
graph->adj_matrix[i][j] = 0;
}
}
// 初始化邻接表
for (int i = 0; i < graph->vertex_num; i++) {
graph->adj_list[i].vertex = i;
graph->adj_list[i].first_edge = NULL;
}
// 输入每条边的信息,并构建邻接矩阵和邻接表
for (int i = 0; i < graph->edge_num; i++) {
printf("请输入第%d条边的起点、终点和权值(用空格隔开):", i + 1);
int start, end, weight;
scanf("%d %d %d", &start, &end, &weight);
graph->adj_matrix[start][end] = weight;
graph->adj_matrix[end][start] = weight;
Edge* edge = (Edge*)malloc(sizeof(Edge));
edge->start = start;
edge->end = end;
edge->weight = weight;
EdgeNode* node = (EdgeNode*)malloc(sizeof(EdgeNode));
node->edge = edge;
node->next = graph->adj_list[start].first_edge;
graph->adj_list[start].first_edge = node;
node = (EdgeNode*)malloc(sizeof(EdgeNode));
node->edge = edge;
node->next = graph->adj_list[end].first_edge;
graph->adj_list[end].first_edge = node;
}
}
```
接下来,我们可以实现图的深度优先搜索遍历和广度优先搜索遍历。这里使用栈和队列来实现:
```c
// 深度优先搜索遍历
void dfs(Graph* graph, int vertex, bool* visited) {
printf("%d ", vertex);
visited[vertex] = true;
for (int i = 0; i < graph->vertex_num; i++) {
if (graph->adj_matrix[vertex][i] != 0 && !visited[i]) {
dfs(graph, i, visited);
}
}
}
void dfsTraversal(Graph* graph) {
bool visited[MAX_VERTEX_NUM] = {false};
for (int i = 0; i < graph->vertex_num; i++) {
if (!visited[i]) {
dfs(graph, i, visited);
}
}
}
// 广度优先搜索遍历
void bfsTraversal(Graph* graph) {
bool visited[MAX_VERTEX_NUM] = {false};
Queue queue;
initQueue(&queue);
for (int i = 0; i < graph->vertex_num; i++) {
if (!visited[i]) {
printf("%d ", i);
visited[i] = true;
enqueue(&queue, i);
while (!isEmpty(&queue)) {
int vertex = dequeue(&queue);
for (EdgeNode* p = graph->adj_list[vertex].first_edge; p != NULL; p = p->next) {
int end = p->edge->end;
if (!visited[end]) {
printf("%d ", end);
visited[end] = true;
enqueue(&queue, end);
}
}
}
}
}
}
```
最后,我们可以在 main 函数中调用这些函数:
```c
int main() {
Graph graph;
createGraph(&graph);
printf("深度优先搜索遍历序列:");
dfsTraversal(&graph);
printf("\n");
printf("广度优先搜索遍历序列:");
bfsTraversal(&graph);
printf("\n");
return 0;
}
```
这样,我们就完成了题目中所要求的内容。完整代码如下:
阅读全文
相关推荐






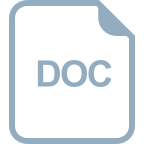









