vue3+js+mock+vite实现ToduList
时间: 2023-05-25 13:04:33 浏览: 135
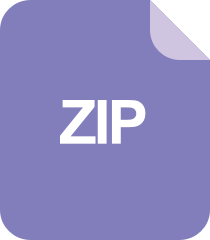
基于VUE3+vite框架搭建的失物招领后台管理系统及数据可视化大屏
作为语言模型,我无法实际编写和运行代码,但我可以向您介绍Vue 3和Vite以及如何使用它们编写ToduList应用程序的步骤。
Vue 3是一个流行的JavaScript框架,用于构建交互式应用程序和用户界面。它具有易于学习和使用的API和一些新功能,例如Composition API和Teleport。
Vite是一个快速的现代构建工具,专为大型Web项目而设计。它提供了快速的开发服务器和即时热更新功能。
现在,让我们开始使用Vue 3和Vite构建ToduList应用程序的步骤。
第一步:安装Vite
使用npm安装Vite
```
npm install -g vite
```
第二步:创建Vue 3项目
通过在终端中运行以下命令,在新的Vue 3项目中使用Vite
```
npm init vite@latest my-todo-list
```
或使用npx安装和创建一个新的Vue 3项目
```
npx create-vite-app my-todo-list --template vue
```
这将创建一个名为' my-todo-list '的新目录并初始化一个基本的Vue 3项目结构。
第三步:安装和配置所需的依赖项
使用以下命令安装axios,用于从后端API获取数据
```
npm install axios
```
在'todo list/src'文件夹里新建一个'Api.js'文件:
```
import axios from 'axios'
const apiClient = axios.create({
baseURL: `http://localhost:8000/`, // change to API URL
headers: {
Accept: 'application/json',
'Content-Type': 'application/json'
}
})
export default {
getTodos() {
return apiClient.get('/todos')
},
editTodoStatus(id, data) {
return apiClient.put(`/todos/${id}`, data)
},
addNewTodo() {
return apiClient.post('/todos')
},
deleteTodoById(id) {
return apiClient.delete(`/todos/${id}`)
}
}
```
第四步:定义应用程序
编辑'todo list/src/App.vue'文件
```
<template>
<div class="container">
<h1 class="text-4xl text-center my-8"> TODO LIST </h1>
<div class="my-8">
<button @click.prevent="addNewTodo" class="bg-blue-500 hover:bg-blue-700 text-white font-bold py-2 px-4 rounded">
Add New Todo
</button>
</div>
<div class="my-8">
<ul>
<li v-for="todo in todos" :key="todo.id" class="mb-4">
<div class="flex justify-between items-center">
<div>
<input type="checkbox" :checked="todo.completed" @change="toggleCompletion(todo)" class="mr-2" />
<span class="font-bold">{{todo.title}}</span>
</div>
<div class="flex">
<button class="bg-red-500 hover:bg-red-700 text-white font-bold py-2 px-4 rounded-lg" @click.prevent="deleteTodoById(todo.id)">
<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 20 20" fill="currentColor" class="h-5 w-5">
<path
fill-rule="evenodd"
d="M14.823 1.77a.75.75 0 01-.06 1.06L10.56 6.25l4.204 4.424a.75.75 0 11-1.12 1.012L9.25 7.812l-4.344 4.575a.75.75 0 01-1.12-1.012L7.93 6.25.765 1.832a.75.75 0 111.06-1.06L9.25 5.688l5.573-5.918a.75.75 0 011.001-.106z"
clip-rule="evenodd"
/>
</svg>
</button>
</div>
</div>
</li>
</ul>
</div>
</div>
</template>
<script>
import Api from '@/Api'
export default {
name: 'App',
data() {
return {
todos: []
}
},
async created() {
await this.fetchData()
},
methods: {
async fetchData() {
const { data: todos } = await Api.getTodos()
this.todos = todos
},
async toggleCompletion(todo) {
await Api.editTodoStatus(todo.id, { completed: !todo.completed })
await this.fetchData()
},
async addNewTodo() {
await Api.addNewTodo()
await this.fetchData()
},
async deleteTodoById(id) {
await Api.deleteTodoById(id)
await this.fetchData()
}
}
}
</script>
<style>
/* ... */
</style>
```
第五步:运行程序
使用以下命令运行程序
```
npm run dev
```
现在在浏览器中访问'http:// localhost:3000'即可看到ToduList应用程序。
可以使用Api.js文件里的接口完成每操作,此处用axios调用后台API进行模拟,并未真正实现后端API。
阅读全文
相关推荐
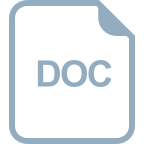
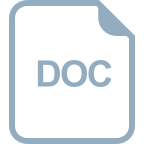
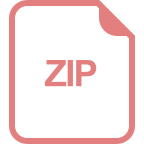
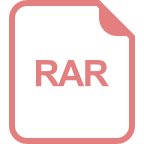
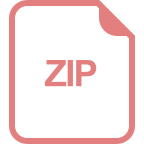
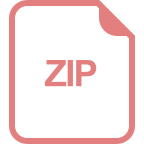



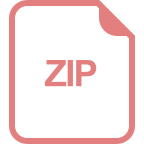
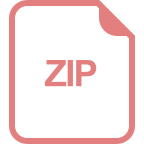
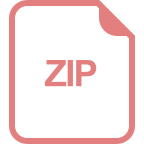
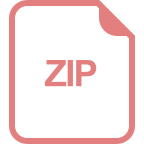
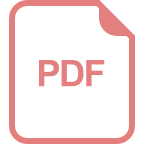
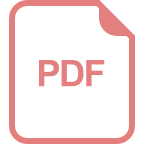
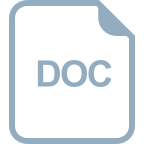
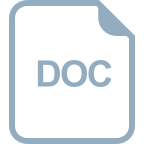
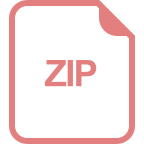