文件管理程序C++
时间: 2023-07-11 13:17:08 浏览: 49
好的,你可以尝试以下的文件管理程序C++代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <dirent.h>
#include <sys/stat.h>
#include <unistd.h>
using namespace std;
void list_files(string path) {
DIR *dir;
struct dirent *ent;
if ((dir = opendir(path.c_str())) != NULL) {
while ((ent = readdir(dir)) != NULL) {
if (ent->d_name[0] != '.') {
cout << ent->d_name << endl;
}
}
closedir(dir);
} else {
cerr << "Could not open directory: " << path << endl;
}
}
void create_file(string path) {
ofstream file(path.c_str());
if (file.is_open()) {
cout << "File created successfully!" << endl;
} else {
cerr << "Could not create file: " << path << endl;
}
file.close();
}
void delete_file(string path) {
if (remove(path.c_str()) == 0) {
cout << "File deleted successfully!" << endl;
} else {
cerr << "Could not delete file: " << path << endl;
}
}
void rename_file(string old_path, string new_path) {
if (rename(old_path.c_str(), new_path.c_str()) == 0) {
cout << "File renamed successfully!" << endl;
} else {
cerr << "Could not rename file: " << old_path << endl;
}
}
void copy_file(string old_path, string new_path) {
ifstream src(old_path.c_str(), ios::binary);
ofstream dst(new_path.c_str(), ios::binary);
dst << src.rdbuf();
if (src.is_open() && dst.is_open()) {
cout << "File copied successfully!" << endl;
} else {
cerr << "Could not copy file: " << old_path << " to " << new_path << endl;
}
src.close();
dst.close();
}
void move_file(string old_path, string new_path) {
if (rename(old_path.c_str(), new_path.c_str()) == 0) {
cout << "File moved successfully!" << endl;
} else {
cerr << "Could not move file: " << old_path << " to " << new_path << endl;
}
}
void get_file_info(string path) {
struct stat file_info;
if (stat(path.c_str(), &file_info) == 0) {
cout << "File name: " << path << endl;
cout << "File size: " << file_info.st_size << " bytes" << endl;
cout << "File permissions: ";
cout << ((file_info.st_mode & S_IRUSR) ? "r" : "-") << ((file_info.st_mode & S_IWUSR) ? "w" : "-") << ((file_info.st_mode & S_IXUSR) ? "x" : "-");
cout << ((file_info.st_mode & S_IRGRP) ? "r" : "-") << ((file_info.st_mode & S_IWGRP) ? "w" : "-") << ((file_info.st_mode & S_IXGRP) ? "x" : "-");
cout << ((file_info.st_mode & S_IROTH) ? "r" : "-") << ((file_info.st_mode & S_IWOTH) ? "w" : "-") << ((file_info.st_mode & S_IXOTH) ? "x" : "-");
cout << endl;
} else {
cerr << "Could not get file info: " << path << endl;
}
}
int main() {
string path;
int choice;
do {
cout << "Please enter the path of the directory: ";
getline(cin, path);
if (path[path.length() - 1] != '/') {
path += '/';
}
cout << "1. List files" << endl;
cout << "2. Create file" << endl;
cout << "3. Delete file" << endl;
cout << "4. Rename file" << endl;
cout << "5. Copy file" << endl;
cout << "6. Move file" << endl;
cout << "7. Get file info" << endl;
cout << "0. Exit" << endl;
cout << "Please enter your choice: ";
cin >> choice;
cin.ignore();
switch (choice) {
case 1:
list_files(path);
break;
case 2:
cout << "Please enter the name of the file to create: ";
getline(cin, path);
create_file(path);
break;
case 3:
cout << "Please enter the name of the file to delete: ";
getline(cin, path);
delete_file(path);
break;
case 4:
string old_path, new_path;
cout << "Please enter the name of the file to rename: ";
getline(cin, old_path);
cout << "Please enter the new name of the file: ";
getline(cin, new_path);
rename_file(old_path, new_path);
break;
case 5:
cout << "Please enter the name of the file to copy: ";
getline(cin, old_path);
cout << "Please enter the name of the new file: ";
getline(cin, new_path);
copy_file(old_path, new_path);
break;
case 6:
cout << "Please enter the name of the file to move: ";
getline(cin, old_path);
cout << "Please enter the new name of the file: ";
getline(cin, new_path);
move_file(old_path, new_path);
break;
case 7:
cout << "Please enter the name of the file to get info: ";
getline(cin, path);
get_file_info(path);
break;
case 0:
break;
default:
cerr << "Invalid choice!" << endl;
break;
}
} while (choice != 0);
return 0;
}
```
这个文件管理程序可以列出指定目录下的所有文件、创建文件、删除文件、重命名文件、复制文件、移动文件和获取文件信息。你可以根据需要进行修改和扩展。
相关推荐
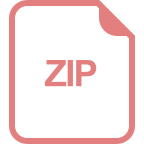
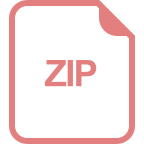
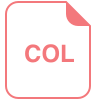
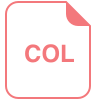
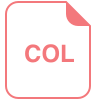
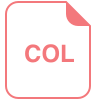
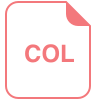









