编写一个程序,可以在命令行输入参数,完成指定文件的缩放,并存储到新文件,命令行参数如下
时间: 2023-12-05 07:03:24 浏览: 33
以下是一个 Python 程序示例,可以在命令行输入参数,完成指定文件的缩放,并存储到新文件:
```python
import sys
from PIL import Image
# 获取命令行参数
if len(sys.argv) != 4:
print("Usage: python resize_image.py [input_file] [output_file] [scale_factor]")
sys.exit(1)
input_file = sys.argv[1]
output_file = sys.argv[2]
scale_factor = float(sys.argv[3])
# 打开图片文件
try:
image = Image.open(input_file)
except IOError:
print("Error: Failed to open image file")
sys.exit(1)
# 计算缩放后的尺寸
width, height = image.size
new_width = int(width * scale_factor)
new_height = int(height * scale_factor)
# 缩放图片
try:
new_image = image.resize((new_width, new_height), Image.ANTIALIAS)
except ValueError:
print("Error: Invalid scale factor")
sys.exit(1)
# 存储缩放后的图片
try:
new_image.save(output_file)
except IOError:
print("Error: Failed to save image file")
sys.exit(1)
print("Image successfully resized and saved to {}".format(output_file))
```
这个程序使用了 Pillow 库(Python Imaging Library 的一个分支),可以使用以下命令安装:
```
pip install Pillow
```
在命令行中运行程序时,需要提供三个参数:输入文件名、输出文件名和缩放因子。例如:
```
python resize_image.py input.jpg output.jpg 0.5
```
这个命令会将 input.jpg 文件缩小为原来的一半,并将结果保存到 output.jpg 文件中。
相关推荐
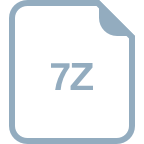
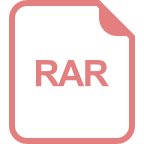
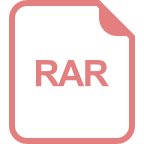










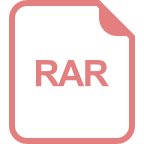
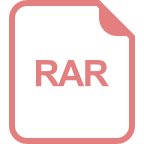
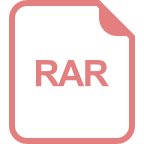
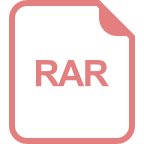
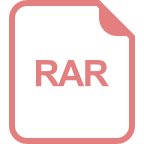