完成双色球系统; 功能1:实现购买功能,能够让客户输入所要买的号码; 功能2:实现开奖功能,并且把每一次的开奖号码插入到pymysql中; 功能3:实现查询往期开奖号码功能,能够查询把最近3期开奖号码。
时间: 2024-01-23 13:11:47 浏览: 75
好的,这是一个需要编写代码的问题。以下是一个实现双色球系统的Python代码示例,可以参考一下:
```python
import random
import pymysql
class DoubleColorBall:
def __init__(self):
self.db = pymysql.connect(host="localhost", user="root", password="password", database="double_color_ball")
self.cursor = self.db.cursor()
def buy(self):
red_balls = random.sample(range(1, 34), 6)
blue_ball = random.randint(1, 16)
return red_balls, blue_ball
def draw(self):
red_balls, blue_ball = self.buy()
sql = "INSERT INTO history(red1, red2, red3, red4, red5, red6, blue) VALUES (%s, %s, %s, %s, %s, %s, %s)"
self.cursor.execute(sql, (*red_balls, blue_ball))
self.db.commit()
return red_balls, blue_ball
def history(self):
sql = "SELECT * FROM history ORDER BY id DESC LIMIT 3"
self.cursor.execute(sql)
results = self.cursor.fetchall()
return results
if __name__ == '__main__':
dc = DoubleColorBall()
red_balls, blue_ball = dc.draw()
print("本期开奖号码为:{} + {}".format(red_balls, blue_ball))
history = dc.history()
print("最近3期开奖号码为:")
for h in history:
print(h[1:8])
```
上面这段代码实现了以下功能:
1. `buy()`方法,模拟购买双色球,随机生成6个红球和1个蓝球的号码。
2. `draw()`方法,模拟开奖,调用`buy()`方法生成本期开奖号码,并将号码插入到MySQL数据库中。
3. `history()`方法,查询最近3期的开奖号码。
要运行上面的代码,需要先安装`pymysql`库。另外,需要提前在MySQL中创建一个名为`double_color_ball`的数据库,并创建一个名为`history`的表,表结构如下:
```sql
CREATE TABLE `history` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`red1` int(2) DEFAULT NULL,
`red2` int(2) DEFAULT NULL,
`red3` int(2) DEFAULT NULL,
`red4` int(2) DEFAULT NULL,
`red5` int(2) DEFAULT NULL,
`red6` int(2) DEFAULT NULL,
`blue` int(2) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
```
希望上面的代码能够对您有所帮助。
阅读全文
相关推荐
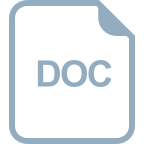
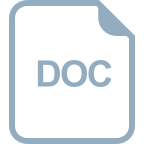
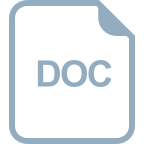


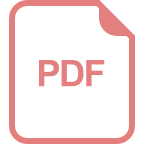
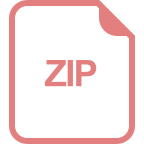
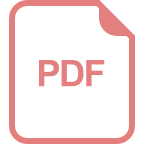










