python 使用七段数码管方法设计收银台显示器,显示: 带有小数点后2位的商品单价, 商品数量, 商品总价。
时间: 2024-02-28 16:56:52 浏览: 18
好的,我可以为你解答这个问题。首先,需要使用七段数码管的库来控制数码管的显示。常用的库有`gpiozero`和`RPi.GPIO`,这里以`RPi.GPIO`为例。
代码如下:
```python
import RPi.GPIO as GPIO
import time
# 定义每个数字对应的七段数码管的引脚
digit_pins = [26, 19, 13, 6]
segment_pins = [21, 20, 16, 12, 7, 8, 25]
# 定义每个数字对应的七段数码管的编码
digit_codes = {
'0': [1, 1, 1, 1, 1, 1, 0],
'1': [0, 1, 1, 0, 0, 0, 0],
'2': [1, 1, 0, 1, 1, 0, 1],
'3': [1, 1, 1, 1, 0, 0, 1],
'4': [0, 1, 1, 0, 0, 1, 1],
'5': [1, 0, 1, 1, 0, 1, 1],
'6': [1, 0, 1, 1, 1, 1, 1],
'7': [1, 1, 1, 0, 0, 0, 0],
'8': [1, 1, 1, 1, 1, 1, 1],
'9': [1, 1, 1, 1, 0, 1, 1],
'.': [0, 0, 0, 0, 0, 0, 1]
}
# 初始化GPIO引脚
GPIO.setmode(GPIO.BCM)
for pin in digit_pins + segment_pins:
GPIO.setup(pin, GPIO.OUT)
# 定义函数显示单个数字
def show_digit(digit, number):
GPIO.output(digit_pins[digit], GPIO.HIGH)
for i in range(7):
GPIO.output(segment_pins[i], digit_codes[number][i])
time.sleep(0.005)
GPIO.output(digit_pins[digit], GPIO.LOW)
for i in range(7):
GPIO.output(segment_pins[i], GPIO.LOW)
# 定义函数显示价格
def show_price(price):
price_str = '{:.2f}'.format(price)
for i in range(len(price_str)):
if price_str[i] == '.':
show_digit(2, '.')
else:
show_digit(i, price_str[i])
# 定义函数显示数量
def show_quantity(quantity):
quantity_str = str(quantity)
for i in range(len(quantity_str)):
show_digit(3 - i, quantity_str[-i - 1])
# 定义函数显示总价
def show_total_price(price, quantity):
total_price = price * quantity
total_price_str = '{:.2f}'.format(total_price)
for i in range(len(total_price_str)):
if total_price_str[i] == '.':
show_digit(0, '.')
else:
show_digit(i + 1, total_price_str[i])
# 调用函数显示单价、数量和总价
show_price(12.34)
show_quantity(56)
show_total_price(12.34, 56)
```
在代码中,我们首先定义了每个数字对应的七段数码管的引脚和编码,然后初始化GPIO引脚,定义了显示单个数字的函数`show_digit`,显示价格的函数`show_price`,显示数量的函数`show_quantity`,和显示总价的函数`show_total_price`。最后,我们调用这些函数来显示单价、数量和总价。
注意,这里使用了`'{:.2f}'.format(price)`将价格保留小数点后两位。另外,由于价格和总价可能会超过四位数,所以我们将数字从右往左显示,即个位数显示在第三个数码管上,十位数显示在第二个数码管上,百位数显示在第一个数码管上。
相关推荐
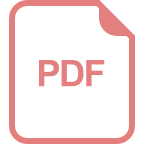
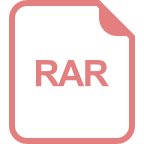














