模板模式 有趣地例子
时间: 2023-06-29 16:12:27 浏览: 49
模板模式是一种行为设计模式,它定义了一个算法的骨架,将一些步骤延迟到子类中实现。这个模式使得子类可以在不改变算法结构的情况下,重新定义算法中的某些步骤。
以下是一个有趣的例子:
假设你是一位烘焙师,你要烤一批饼干。你的烘焙流程如下:
1. 准备材料(面粉、糖、鸡蛋、黄油等)
2. 将材料混合在一起
3. 将混合好的面团放入冰箱冷藏
4. 将面团取出,切成小块
5. 将小块面团放入烤箱烤制
6. 取出烤好的饼干,放凉
这个流程可以使用模板模式来实现。首先定义一个抽象类,包含烤饼干的流程:
```
abstract class CookieRecipe {
public void bakeCookies() {
prepareIngredients();
mixIngredients();
chillDough();
rollDough();
bakeCookiesInOven();
coolCookies();
}
abstract void prepareIngredients();
abstract void mixIngredients();
void chillDough() {
System.out.println("Chilling dough in refrigerator");
}
void rollDough() {
System.out.println("Rolling dough into small balls");
}
void bakeCookiesInOven() {
System.out.println("Baking cookies in the oven");
}
void coolCookies() {
System.out.println("Cooling cookies on rack");
}
}
```
然后,我们可以创建一个具体子类来实现每个步骤:
```
class ChocolateChipCookieRecipe extends CookieRecipe {
void prepareIngredients() {
System.out.println("Preparing chocolate chip cookie ingredients...");
}
void mixIngredients() {
System.out.println("Mixing chocolate chip cookie ingredients...");
}
}
class OatmealRaisinCookieRecipe extends CookieRecipe {
void prepareIngredients() {
System.out.println("Preparing oatmeal raisin cookie ingredients...");
}
void mixIngredients() {
System.out.println("Mixing oatmeal raisin cookie ingredients...");
}
}
```
现在,我们可以使用这些子类来烤出不同种类的饼干:
```
CookieRecipe recipe = new ChocolateChipCookieRecipe();
recipe.bakeCookies(); // 烤出巧克力曲奇
recipe = new OatmealRaisinCookieRecipe();
recipe.bakeCookies(); // 烤出燕麦葡萄干曲奇
```
模板模式使得我们可以定义一个通用的烤饼干流程,然后在子类中实现具体的步骤。这种方式可以帮助我们避免重复代码并提高代码的可维护性。
相关推荐
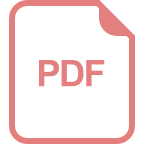
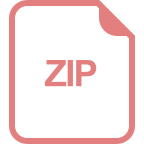
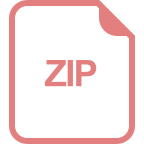
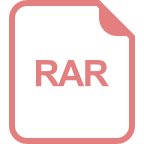
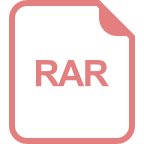
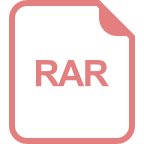
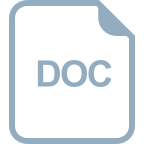
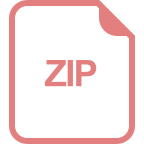
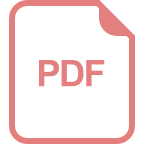
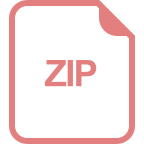
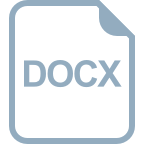
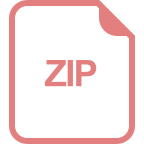
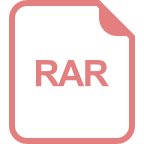
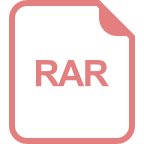