将以下程序段翻译成四元式序列。 While a>0 ∨b<0 do Begin X=X+1; if a>0 then a=a-1 else b=b+1 End;
时间: 2023-10-04 22:14:33 浏览: 199
1. (LABEL, _, _, L1)
2. (LOAD, a, _, T1)
3. (JLE, T1, 0, L2)
4. (LABEL, _, _, L3)
5. (LOAD, X, _, T2)
6. (ADD, T2, 1, T3)
7. (STORE, T3, _, X)
8. (LOAD, a, _, T4)
9. (JLE, T4, 0, L4)
10. (LOAD, a, _, T5)
11. (SUB, T5, 1, T6)
12. (STORE, T6, _, a)
13. (JUMP, _, _, L5)
14. (LABEL, _, _, L4)
15. (LOAD, b, _, T7)
16. (ADD, T7, 1, T8)
17. (STORE, T8, _, b)
18. (LABEL, _, _, L5)
19. (LOAD, a, _, T9)
20. (JGT, T9, 0, L3)
21. (LABEL, _, _, L2)
相关问题
用C++实现下面要求(1)关键字 main if else int return void while 所有的关键字都是小写。 (2)专用符号 = + - * / < < = > > = = = != ; : , { } [ ] ( ) (3)其他标记ID和NUM 通过以下正规式定义其他标记: ID letter(letter | didit)* NUM digit digit* letter a |…| z | A|…|Z digit 0|…| 9 (4)空格有空白、制表符和换行符组成 空格一般用来分隔ID、NUM、专用符号和关键字,词法分析阶段通常被忽略。 2.2各种单词符号对应的种别码 表C.1 各种单词符号的种别码 单词符号 种别码 单词符号 种别码 main 1 [ 28 int 2 ] 29 char 3 { 30 if 4 } 31 for 5 , 32 else 6 : 33 while 7 ; 34 ID 10 > 35 NUM 20 < 36 = 21 >= 37 + 22 <= 38 - 23 == 39 * 24 != 40 / 25 ‘\0’ 1000 ( 26 ERROR -1 ( 27 2.3 词法分析程序的功能 输入:所给文法的原程序字符串。 输出:二元组(syn,token或sum)构成的序列。其中, ●syn为单词种别码。 ●token为存放的单词自身字符串。 ● sum为整型常量。 具体实现时,可以将单词的二元组用结构进行处理。 例如,对源程序 main( ) { int i=10; while( i ) i=i-1; } 的源文件,经词法分析后输出如下序列: (1,main)(26,( ) (27,) ) (30,{) (2,int) (10,i) (21,=) (20,10) (34, ;) (7,while) (26,( ) (10,i) (27,) ) (10,i) (21,=) (10,i) (23,-) (20,1) (34, ;) (31, })
以下是一个基于C++实现的简单词法分析程序,能够识别给定的C语言子集。该程序使用了正则表达式库regex来匹配单词。具体实现中,使用了一个Token结构体来存储识别出的单词及其种别码和值。
```c++
#include <iostream>
#include <string>
#include <regex>
using namespace std;
// Token结构体
struct Token {
int syn; // 单词种别码
string token; // 单词字符串
int value; // 整数值
};
int main() {
// 正则表达式
regex keywords("main|if|else|int|return|void|while");
regex symbols("[=+\\-*/<>]=|!=|==|&&|\\|\\||[{}\\[\\]();,:]");
regex id("[a-zA-Z][a-zA-Z0-9]*");
regex num("[0-9]+");
// 待匹配的字符串
string code = "main() {\n int i = 10;\n while(i) {\n i = i - 1;\n }\n}";
// 用于存储识别出的单词序列
vector<Token> tokens;
// 逐个匹配字符串中的单词
for (sregex_iterator it(code.begin(), code.end(), keywords), end_it; it != end_it; ++it) {
Token token;
token.syn = 1;
token.token = it->str();
tokens.push_back(token);
}
for (sregex_iterator it(code.begin(), code.end(), symbols), end_it; it != end_it; ++it) {
Token token;
string symbol = it->str();
if (symbol == "+") token.syn = 22;
else if (symbol == "-") token.syn = 23;
else if (symbol == "*") token.syn = 24;
else if (symbol == "/") token.syn = 25;
else if (symbol == "<") token.syn = 36;
else if (symbol == "<=") token.syn = 38;
else if (symbol == ">") token.syn = 35;
else if (symbol == ">=") token.syn = 37;
else if (symbol == "==") token.syn = 39;
else if (symbol == "!=") token.syn = 40;
else if (symbol == ";") token.syn = 34;
else if (symbol == "{") token.syn = 30;
else if (symbol == "}") token.syn = 31;
else if (symbol == "[") token.syn = 28;
else if (symbol == "]") token.syn = 29;
else if (symbol == "(") token.syn = 26;
else if (symbol == ")") token.syn = 27;
else if (symbol == ",") token.syn = 32;
else if (symbol == ":") token.syn = 33;
token.token = symbol;
tokens.push_back(token);
}
for (sregex_iterator it(code.begin(), code.end(), id), end_it; it != end_it; ++it) {
Token token;
token.syn = 10;
token.token = it->str();
tokens.push_back(token);
}
for (sregex_iterator it(code.begin(), code.end(), num), end_it; it != end_it; ++it) {
Token token;
token.syn = 20;
token.token = it->str();
token.value = stoi(it->str());
tokens.push_back(token);
}
// 输出识别出的单词序列
for (auto t : tokens) {
cout << "(" << t.syn << "," << t.token;
if (t.syn == 20) {
cout << "," << t.value;
}
cout << ") ";
}
cout << endl;
return 0;
}
```
该程序将给定的C语言子集分为关键字、符号、标识符和数字四类单词,使用Token结构体存储每个识别出的单词,并输出识别出的单词序列。
void analyseAnagram(const vector<string> & dictionary) { cout << "请输入单词(或任意字母序列)" << endl; for (string_input p(cin); p != string_input(); ++p) { cout << "查找输入单词的变位词中..." << endl; string word = *p; sort(word.begin(), word.end()); bool found_one = false; do { if (binary_search(dictionary.begin(), dictionary.end(), word)) { cout << " " << word ; found_one = true; } } while (next_permutation(word.begin(), word.end())); if (!found_one) cout << " 抱歉,没有找到变位词\n"; cout << "\n请输入下一个单词 " << "(或输入Ctrl+Z终止程序 ) \n" << endl; } }
这段代码实现了一个函数`analyseAnagram`,它接受一个字符串向量`dictionary`作为参数,并且通过不断读入用户输入的单词或字母序列,查找其在`dictionary`中的变位词并输出。具体实现如下:
1. 首先输出提示信息,让用户输入要查找的单词。
2. 进入循环,不断读入用户的输入单词或字母序列。
3. 对于每个输入单词,先将其排序,然后通过`binary_search`函数在`dictionary`中查找其变位词是否存在。
4. 如果找到了变位词,则输出变位词;否则输出提示信息表明没有找到。
5. 输出提示信息,让用户输入下一个单词,继续循环。
6. 如果用户输入了Ctrl+Z,则程序终止。
需要注意的是,这段代码使用了标准库中的`sort`函数和`binary_search`函数,以及`next_permutation`函数来生成一个字符串的所有排列。同时,它还重载了输入流运算符`>>`来读入用户的输入。
阅读全文
相关推荐
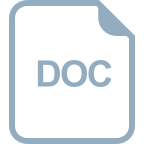
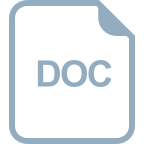
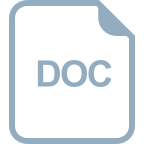



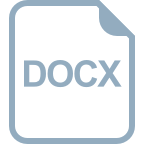
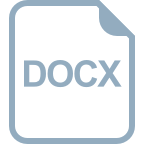
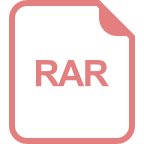
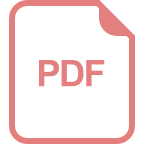
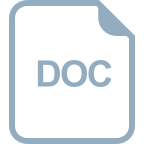




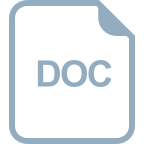