以下代码在曾经调试过程中可能出现的问题#include <iostream> #include <fstream> #include <string> #include <vector> #include <algorithm> #include <iterator> using namespace std; typedef istream_iterator<string> string_input; void welcome() { cout << "******************* 变位词查找系统*********************\n" << "在词典中找出给定的字符串的所有变位词" << endl; } void readDict(vector<string> & dictionary) { cout << "首先,请输入词典的文件名称:" << endl; string dictionary_name; cin >> dictionary_name; ifstream ifs(dictionary_name.c_str()); if (!ifs.is_open()) { cerr << "异常:文件"<< dictionary_name << "没有找到 " << endl; exit(1); } cout << "词典读入中 ..." << flush; copy(string_input(ifs), string_input(), back_inserter(dictionary)); sort(dictionary.begin(),dictionary.end()); cout << "词典包含有 " << dictionary.size() << " 个单词\n\n"; ifs.close(); } void analyseAnagram(const vector<string> & dictionary) { cout << "请输入单词(或任意字母序列)" << endl; for (string_input p(cin); p != string_input(); ++p) { cout << "查找输入单词的变位词中..." << endl; string word = *p; sort(word.begin(), word.end()); bool found_one = false; do { if (binary_search(dictionary.begin(), dictionary.end(), word)) { cout << " " << word ; found_one = true; } } while (next_permutation(word.begin(), word.end())); if (!found_one) cout << " 抱歉,没有找到变位词\n"; cout << "\n请输入下一个单词 " << "(或输入Ctrl+Z终止程序 ) \n" << endl; } } int main() { welcome(); vector<string> dictionary; readDict(dictionary); analyseAnagram(dictionary); system("pause"); return 0; }
时间: 2024-04-22 18:22:49 浏览: 61
这段代码可能出现的问题包括:
1. 文件读取问题:如果输入的词典文件名称不存在或者无法打开,程序会抛出异常并退出。
2. 内存问题:如果读入的词典文件太大,可能会导致内存不足,程序崩溃。
3. 输入问题:如果输入的单词中包含非字母的字符,程序可能会出现不可预期的行为。
4. 死循环问题:如果输入的单词中没有任何一个单词是词典中的变位词,程序会进入死循环,无法终止。
5. 输出问题:程序输出的结果可能会过长,导致控制台或者输出文件无法显示完全。
相关问题
#include <iostream> #include <fstream> #include <cstdio> #include <cstdlib> #include <cstring> #include <cmath> #include <climits> #include <string> #include <algorithm> #include <vector> #include <map> using namespace std; struct Value { char str[10000]; }; void structSort(Value *a, int n) { @你的代码 } int n; Value a[5000]; int main() { scanf("%d", &n); for (int i = 0; i<n; i++) { scanf("%s", a[i].str); } structSort(a, n); for (int i = 0; i<n; i++) { printf("%s\n", a[i].str); } return 0; }
这段代码给出了一个结构体数组 `a`,其中每个结构体包含一个字符串成员 `str`。接下来有一个函数 `structSort`,它需要你来实现。你需要使用任何一种排序算法对 `a` 数组进行排序,排序的依据是字符串的字典序(即按照字母顺序进行比较)。最后将排序后的字符串数组依次输出即可。
以下是可能的 `structSort` 实现方式之一,使用了标准库中的 `qsort` 函数:
```c++
int compare(const void *a, const void *b) {
return strcmp(((Value *)a)->str, ((Value *)b)->str);
}
void structSort(Value *a, int n) {
qsort(a, n, sizeof(Value), compare);
}
```
其中,`compare` 函数用于比较两个字符串的大小,将其作为参数传递给 `qsort` 函数进行排序。
我的头文件是这样写的#include <stdio.h> #include <math.h> #include <fstream> #include <iostream> #include <string>//getline #include <sstream> #include "stb_image.h" #pragma comment(lib, "openGL32.lib") #pragma comment(lib, "glu32.lib") #pragma comment(lib, "glaux.lib") #include <gl/glaux.h> #include "glew.h" #include "glut.h" #include <vector>,请问如何避免报错gl.h included before glew.h呢
在你的头文件中,应该将 `glew.h` 的引入放在 `gl.h` 的引入之前。因此,你应该将 `glew.h` 的引入语句移到 `gl.h` 的引入语句之前,就像这样:
```
#include <stdio.h>
#include <math.h>
#include <fstream>
#include <iostream>
#include <string>//getline
#include <sstream>
#include "stb_image.h"
#include <gl/glaux.h>
#include <vector>
#include "glew.h"
#include <gl/glut.h>
#pragma comment(lib, "openGL32.lib")
#pragma comment(lib, "glu32.lib")
#pragma comment(lib, "glaux.lib")
```
这样就能够避免 `gl.h included before glew.h` 的错误了。
阅读全文
相关推荐
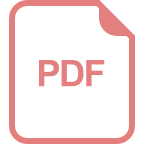
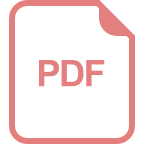
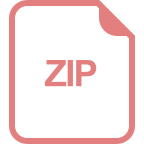














