#include <stdio.h> #include <stdlib.h> void maxmin(int A[],int l,int r,int *maxval,int *minval) { } int main() { int maxv,minv; int A[100]; int i,n; scanf("%d",&n); for(i=0;i<n;i++) { scanf("%d",&A[i]); } maxmin(A,0,n-1,&maxv,&minv); printf("%d %d",maxv,minv); return 0; }
时间: 2023-12-06 14:41:19 浏览: 92
这段代码定义了一个函数 `maxmin`,它的作用是找出数组 `A` 在区间 `[l,r]` 中的最大值和最小值,并通过指针返回给调用者。在主函数中,通过读入数组元素,调用 `maxmin` 函数,输出最大值和最小值。
然而,函数体中并没有给 `maxval` 和 `minval` 赋值,需要在函数体中添加相应的代码来实现找出最大值和最小值的功能。
相关问题
#include <stdio.h> #include <stdlib.h> #define ROW 6 #define COL 6 int main() { int score[ROW][COL]; int cards[6]; int i, j, k, num, dir, total_score; // input score table for (i = 0; i < ROW; i++) { for (j = 0; j < COL; j++) { scanf("%d", &score[i][j]); } } // input cards for (i = 0; i < 6; i++) { scanf("%d", &cards[i]); } // input and process each customer for (k = 0; k < 3; k++) { total_score = 0; scanf("%d %d", &num, &dir); // calculate score if (cards[num-1] == 0) { printf("Invalid card number!\n"); continue; } if (dir == 1) { for (i = 0; i < COL; i++) { total_score += score[num-1][i]; } } else if (dir == 2) { for (i = 0; i < ROW; i++) { total_score += score[i][num-1]; } } else { printf("Invalid direction!\n"); continue; } printf("%d\n", total_score); } return 0; } 改进上述代码
上述代码已经可以正常运行,但是可以进行一些改进:
1. 可以增加注释,让代码更易读懂。
2. 可以在输入时对输入数据进行合法性检查,比如积分表中的数字应该在1~100之间,卡牌数字应该在0~6之间,等等。
3. 可以将代码拆分成多个函数,让主函数更加简洁明了。
4. 可以增加错误处理机制,比如输入数据格式错误时给出提示,让程序更加健壮。
下面是改进后的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define ROW 6
#define COL 6
// 检查输入的数字是否合法,若不合法则返回0,否则返回1
int isValidNumber(int num, int min, int max)
{
if (num < min || num > max)
{
return 0;
}
return 1;
}
// 读取积分表
void readScoreTable(int score[ROW][COL])
{
int i, j;
printf("Please input the score table:\n");
for (i = 0; i < ROW; i++)
{
for (j = 0; j < COL; j++)
{
scanf("%d", &score[i][j]);
if (!isValidNumber(score[i][j], 1, 100))
{
printf("Invalid input: score should be between 1 and 100!\n");
exit(1);
}
}
}
}
// 读取卡牌
void readCards(int cards[6])
{
int i;
printf("Please input the cards:\n");
for (i = 0; i < 6; i++)
{
scanf("%d", &cards[i]);
if (!isValidNumber(cards[i], 0, 6))
{
printf("Invalid input: card number should be between 0 and 6!\n");
exit(1);
}
}
}
// 计算得分
int calculateScore(int score[ROW][COL], int cards[6], int num, int dir)
{
int i, total_score = 0;
if (cards[num-1] == 0)
{
printf("Invalid input: card number %d is hidden!\n", num);
return 0;
}
if (dir == 1)
{
for (i = 0; i < COL; i++)
{
total_score += score[num-1][i];
}
}
else if (dir == 2)
{
for (i = 0; i < ROW; i++)
{
total_score += score[i][num-1];
}
}
else
{
printf("Invalid input: direction should be 1 or 2!\n");
return 0;
}
return total_score;
}
// 处理每个顾客的输入和输出
void processCustomer(int score[ROW][COL], int cards[6])
{
int i, num, dir, total_score;
for (i = 0; i < 3; i++)
{
printf("Please input the card number and direction for customer %d:\n", i+1);
scanf("%d %d", &num, &dir);
total_score = calculateScore(score, cards, num, dir);
printf("Customer %d's score is %d\n", i+1, total_score);
}
}
int main()
{
int score[ROW][COL];
int cards[6];
readScoreTable(score);
readCards(cards);
processCustomer(score, cards);
return 0;
}
```
指出下面代码的问题 运行出现了段错误 #include <openssl/ssl.h> #include <openssl/bio.h> #include <stdio.h> #include <stdlib.h> #include <unistd.h> #include <string.h> #include <netinet/in.h> #include <netdb.h> #include <sys/socket.h> #include <openssl/err.h> void parse_url(char *url, char *protocol, char *domain, char path) { char ptr; if (strncmp(url, "http://", 7) == 0) { strcpy(protocol, "http"); ptr = url + 7; } else if (strncmp(url, "https://", 8) == 0) { strcpy(protocol, "https"); ptr = url + 8; } else { strcpy(protocol, ""); ptr = url; } char domain_end = strstr(ptr, "/"); if (domain_end == NULL) { strcpy(domain, ptr); strcpy(path, ""); } else { int len = domain_end - ptr; strncpy(domain, ptr, len); domain[len] = '\0'; strcpy(path, domain_end); } } int https_communication(char url, char message, char response) { int sockfd, err; struct sockaddr_in serv_addr; struct hostent *server; SSL_CTX *ctx; SSL *ssl; char buf[1024]; printf("test1111 " ); // 创建socket sockfd = socket(AF_INET, SOCK_STREAM, 0); if (sockfd < 0) { perror("ERROR opening socket"); return -1; } // 获取主机信息 server = gethostbyname(url); printf("test1111 " ); if (server == NULL) { perror("ERROR, no such host"); return -1; } // 设置服务地址 printf("test1111 " ); bzero((char *) &serv_addr, sizeof(serv_addr)); serv_addr.sin_family = AF_INET; bcopy((char *)server->h_addr, (char *)&serv_addr.sin_addr.s_addr, server->h_length); serv_addr.sin_port = htons(443); printf("test222 " ); // 连接服务器 if (connect(sockfd,(struct sockaddr *) &serv_addr,sizeof(serv_addr)) < 0) { perror("ERROR connecting"); return -1; } // 初始化SSL SSL_library_init(); ctx = SSL_CTX_new(TLS_method()); if (ctx == NULL) { perror("SSL_CTX_new"); return (-1); } // 设置支持的协议版本为 TLSv1.2 SSL_CTX_set_min_proto_version(ctx, TLS1_2_VERSION); SSL_CTX_set_max_proto_version(ctx, TLS1_2_VERSION); //ctx = SSL_CTX_new(TLSv1_2_client_method()); if (ctx == NULL) { perror("ERROR creating SSL context"); return -1; } printf("test1111 " ); // 创建SSL套接字 ssl = SSL_new(ctx); SSL_set_fd(ssl, sockfd); // SSL握手 err = SSL_connect(ssl); if (err < 0) { perror("ERROR performing SSL handshake"); return -1; } printf("test1111 " ); // 发送消息 char request[1024]; sprintf(request, "GET %s HTTP/1.1\r\nHost: %s\r\n\r\n", message, url); err = SSL_write(ssl, request, strlen(request)); if (err < 0) { perror("ERROR writing to socket"); return -1; } printf("test1111 " ); // 接收响应 bzero(buf, sizeof(buf)); err = SSL_read(ssl, buf, sizeof(buf)-1); if (err < 0) { perror("ERROR reading from socket"); return -1; } printf("test1111999999999 " ); strcpy(response, buf); // 关闭SSL套接字 SSL_free(ssl); SSL_CTX_free(ctx); // 关闭socket close(sockfd); return 0; } int main() { //char *url = "https://otaapi-sit.lotuscars.com.cn/v50/vehicle/api/register"; //char protocol[10], domain[100], path[1000]; //parse_url(url, protocol, domain, path); //printf("protocol: %s\n", protocol); //printf("domain: %s\n", domain); //printf("path: %s\n", path); char *url = "otaapi-sit.lotuscars.com.cn"; //const char *cert_path="/home/pi/Cdaima/certDHU.pem"; char *payload= "{"timestamp":1685523498,"sdkVersion":"1.0.30_1","vin":"LJUBMSA24PKFFF198","sign":"696c3034f04f2bd80c8929089a0deea8cf4812574b002a555f8af663e500c4ce"}"; char *response=NULL; //https_request(cert_path,payload,url) ; //gethostbyname(url); https_communication(url, payload, response); return 0; }
这段代码存在以下问题:
1. 函数声明和调用中的参数类型错误,url, message, response应该是字符数组类型,而不是字符类型。
2. parse_url函数中的path参数类型应该是字符数组类型而不是字符类型。
3. parse_url函数中的domain_end变量应该是字符指针类型而不是字符类型。
4. parse_url函数中的path参数应该使用strcpy函数而不是赋值操作。
5. https_communication函数中的response指针没有被初始化,应该先为其分配内存。
6. https_communication函数中的payload参数类型应该是字符指针类型而不是字符类型。
7. https_communication函数中的buf数组可能存在溢出问题,应该使用sizeof(buf)-1来避免。
8. 在https_communication函数中,SSL_library_init()应该在SSL_CTX_new()之前调用。
阅读全文
相关推荐
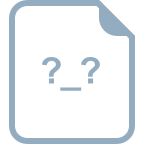
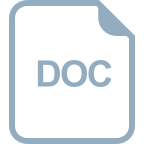













