用C语言数组实现实验: 建立一个记录有序表并实现其上的折半查找。 实验要求: (1) 应用线性表的顺序表实现一个记录有序表; (2) 编码实现在有序表中折半查找某个元素;
时间: 2024-12-16 22:29:40 浏览: 1
在C语言中,可以使用结构体表示记录,并通过动态内存分配创建一个动态大小的顺序表来实现记录有序表。下面是一个简单的步骤和示例代码:
首先,定义一个结构体(如`Record`),它包含你要存储的数据和其他必要的属性:
```c
typedef struct Record {
int data; // 记录数据域
struct Record* next; // 指向下一个记录的指针
} Record;
```
接下来,编写函数来创建、插入和排序记录以及执行折半查找:
```c
// 创建一个新的有序表节点
Record* create_record(int data) {
Record* new_node = (Record*)malloc(sizeof(Record));
if (new_node != NULL) {
new_node->data = data;
new_node->next = NULL;
}
return new_node;
}
// 插入新记录保持有序
void insert_sorted(Record** head, int data) {
Record* current = *head;
Record* prev = NULL;
while (current && current->data < data) {
prev = current;
current = current->next;
}
if (prev == NULL) {
*head = create_record(data);
} else {
prev->next = create_record(data);
}
}
// 折半查找
Record* binary_search(Record* head, int target) {
Record* start = head;
Record* end = head;
while (start != end) {
int mid = (start->data + end->data) / 2;
if (start->data <= target && target < end->data) {
for (int i = 0; i < (end - start); ++i) {
if (start[i].data == target) {
return &start[i];
}
}
// 如果target恰好在中间位置,返回mid
if (start[i].data == mid) {
return &start[i];
}
// 否则,在左半部分继续查找
start = start + (end - start) / 2;
} else if (target < start->data) {
end = start;
} else {
start = start + 1;
}
}
// 没有找到目标,返回NULL
return NULL;
}
```
实验要求:
1. 使用`create_record`创建新的记录,并通过`insert_sorted`将它们按顺序添加到列表中。
2. 调用`binary_search`函数,传入有序表的头节点和目标值,如果找到该元素,则返回指向它的记录节点。
完成以上代码后,你可以测试这个有序表的折半查找功能是否正常工作。
阅读全文
相关推荐
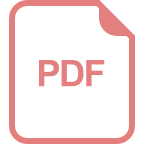
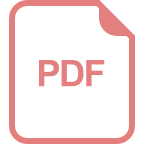
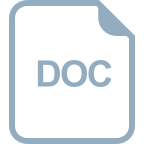
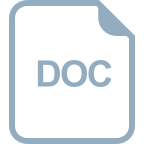
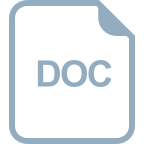
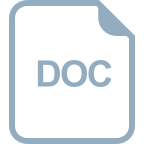
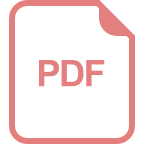
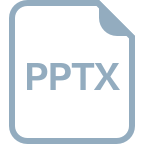
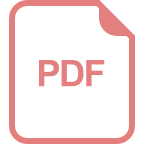
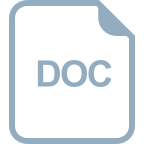
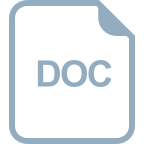
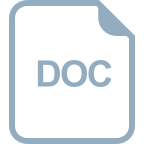
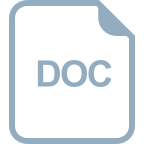
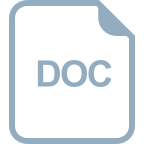
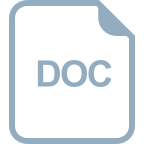
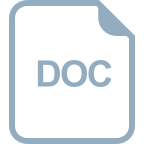
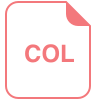