写一段python代码 要求爬取有关“天津机场不明飞行物”的舆论数据 并清洗
时间: 2024-12-20 12:30:24 浏览: 3
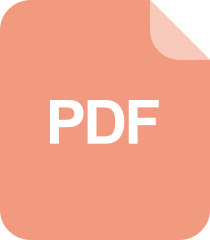
Python爬取数据并实现可视化代码解析
要编写一个Python程序来抓取关于“天津机场不明飞行物”舆情的数据并进行初步清洗,我们需要使用网络爬虫库如`requests`、`BeautifulSoup`或更现代的`pandas`和`selenium`(如果数据来自动态加载的内容)。这里我将提供一个基本的步骤,假设我们使用`requests`和`BeautifulSoup`抓取静态网页上的数据:
```python
import requests
from bs4 import BeautifulSoup
import pandas as pd
# 设置URL
url = "https://www.example.com/search?q=天津机场不明飞行物" # 这里换成实际包含搜索结果的网站URL
# 发送请求
response = requests.get(url)
# 检查请求是否成功
if response.status_code == 200:
# 使用BeautifulSoup解析HTML
soup = BeautifulSoup(response.text, 'html.parser')
# 找到包含评论或新闻的部分(这取决于网站结构)
data_container = soup.find('div', class_='comments') or soup.find('section', id='news_results')
# 假设每个评论是一个字典,有标题(title)、时间(timestamp)和内容(content)字段
comments_list = []
for item in data_container.find_all('article'):
title = item.h2.text.strip() if item.h2 else ""
timestamp = item.time['datetime'] if item.time else ""
content = item.p.text.strip() if item.p else ""
comments_list.append({'Title': title, 'Timestamp': timestamp, 'Content': content})
# 将数据转换为DataFrame
df = pd.DataFrame(comments_list)
# 清洗数据
df['Timestamp'] = pd.to_datetime(df['Timestamp']) # 将时间戳转换为日期时间类型
df.dropna(inplace=True) # 删除缺失值
df['Content'] = df['Content'].str.replace('[^\w\s]', '') # 去除特殊字符
print("清洗后的数据:")
print(df)
else:
print(f"无法访问页面,状态码:{response.status_code}")
```
注意:这段代码示例假设了网站结构以及数据的位置。实际应用时,你需要根据目标网站的具体HTML结构来调整代码。同时,某些网站可能有反爬机制或API接口,直接抓取可能不被允许,请遵守网站的robots.txt规则。
阅读全文
相关推荐
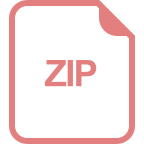
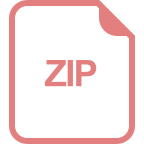



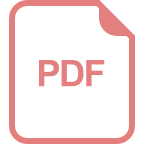
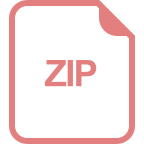
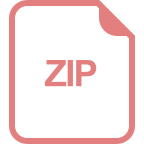
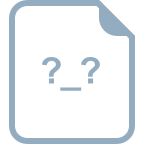





