python爬取数据代码
时间: 2023-10-10 17:03:19 浏览: 195
Python爬取数据的代码可以使用第三方库如BeautifulSoup、Scrapy等来实现。下面是一个简单的示例代码:
```python
import requests
from bs4 import BeautifulSoup
# 发送HTTP请求,获取网页内容
url = 'https://example.com' # 替换为目标网站的URL
response = requests.get(url)
content = response.text
# 使用BeautifulSoup解析HTML
soup = BeautifulSoup(content, 'html.parser')
# 获取需要爬取的数据
data = soup.find('div', class_='data') # 根据实际情况选择合适的标签和属性
# 处理爬取到的数据
result = data.text.strip() # 清除多余的空格和换行符
# 输出结果
print(result)
```
以上代码主要包括三个步骤:
1. 使用`requests`库发送HTTP请求,获取目标网站的HTML内容。
2. 使用`BeautifulSoup`库解析HTML内容,获取需要爬取的数据。
3. 对爬取到的数据进行处理,如清除多余的空格和换行符,并输出结果。
当然,实际的爬取过程通常会更为复杂,可能涉及到网站的登录、滚动加载、反爬机制等。对于这些情况,可能需要结合其他的库或技术来解决。
相关问题
提供一个Python爬取数据的代码
当然可以,以下是一个简单的Python爬虫代码示例:
```python
import requests
from bs4 import BeautifulSoup
url = 'https://www.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
data = soup.find_all('div', {'class': 'example-class'})
for item in data:
print(item.text)
```
这个代码可以爬取一个网站上所有class为example-class的div标签的文本内容。当然,具体的爬取方式还需要根据实际情况进行调整。
python爬取网页数据代码
以下是一个简单的Python爬取网页数据的示例代码:
```python
import requests
# 发送请求
response = requests.get('https://www.example.com')
# 获取响应内容
content = response.content
# 打印响应内容
print(content)
```
以上代码使用了`requests`库向`https://www.example.com`发送了一个GET请求,并获取了响应内容。你可以根据自己的需求对代码进行修改和调整。
阅读全文
相关推荐
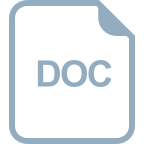
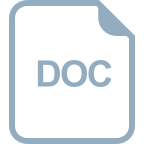
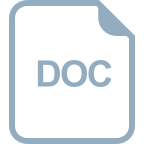
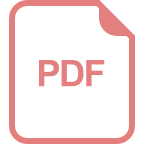
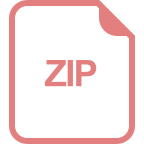
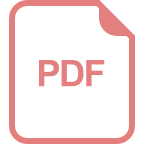
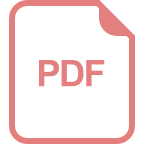
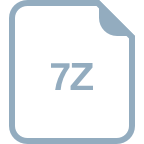
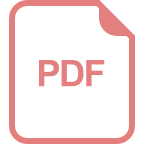
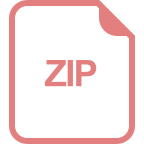






