c++getstrings
时间: 2023-10-28 09:24:38 浏览: 57
c函数getstrings用于获取字符串数组的所有元素。它接受一个字符串数组作为参数,并返回一个指向字符串数组的指针。
以下是一个示例:
```c
#include <stdio.h>
char** getstrings(char** strings, int size) {
return strings;
}
int main() {
char* strings[] = {"Hello", "World", "C", "Programming"};
int size = sizeof(strings) / sizeof(strings[0]);
char** result = getstrings(strings, size);
for (int i = 0; i < size; i++) {
printf("%s\n", result[i]);
}
return 0;
}
```
在上面的示例中,getstrings函数接受字符串数组strings和数组大小size作为参数,并直接返回了该数组。然后,在主函数中调用getstrings函数,并使用循环打印出所有字符串数组的元素。
请注意,此示例中的getstrings函数只是简单地返回给定的字符串数组,并没有进行任何处理或转换。你可以根据实际需求在该函数中添加你需要的逻辑。
相关问题
c++ openssl生成证书
在 C++ 中使用 OpenSSL 生成证书可以分为以下几个步骤:
1. 初始化 OpenSSL 库:
```c++
#include <openssl/ssl.h>
#include <openssl/err.h>
// 初始化 OpenSSL 库
SSL_library_init();
SSL_load_error_strings();
OpenSSL_add_all_algorithms();
```
2. 生成证书请求:
```c++
// 创建 X509_REQ 对象
X509_REQ* req = X509_REQ_new();
// 添加公钥到证书请求
EVP_PKEY* pkey = EVP_PKEY_new();
RSA* rsa = RSA_generate_key(2048, RSA_F4, NULL, NULL);
EVP_PKEY_assign_RSA(pkey, rsa);
X509_REQ_set_pubkey(req, pkey);
// 添加主题信息
X509_NAME* name = X509_NAME_new();
X509_NAME_add_entry_by_NID(name, NID_commonName, MBSTRING_UTF8, (unsigned char*)"example.com", -1, -1, 0);
X509_REQ_set_subject_name(req, name);
// 生成证书请求
X509_REQ_sign(req, pkey, EVP_sha256());
```
3. 生成自签名证书:
```c++
// 创建 X509 对象
X509* cert = X509_new();
// 设置证书版本和序列号
ASN1_INTEGER_set(X509_get_serialNumber(cert), 1);
X509_gmtime_adj(X509_get_notBefore(cert), 0);
X509_gmtime_adj(X509_get_notAfter(cert), 31536000L);
// 设置证书请求信息
X509_set_subject_name(cert, X509_REQ_get_subject_name(req));
X509_set_issuer_name(cert, X509_REQ_get_subject_name(req));
X509_set_pubkey(cert, X509_REQ_get_pubkey(req));
// 签名证书
X509_sign(cert, pkey, EVP_sha256());
```
4. 保存证书和私钥:
```c++
// 保存证书
FILE* fp = fopen("cert.pem", "w");
PEM_write_X509(fp, cert);
fclose(fp);
// 保存私钥
fp = fopen("key.pem", "w");
PEM_write_PrivateKey(fp, pkey, NULL, NULL, 0, NULL, NULL);
fclose(fp);
```
注意:在实际使用中,应该对私钥文件进行保护,例如设置只有 root 用户可读写。
私钥sm2解密 c++
要在 C++ 中解密 SM2 密钥对的私钥,你可以使用 OpenSSL 库提供的函数和工具。下面是一个使用 OpenSSL 解密 SM2 私钥的示例代码:
```cpp
#include <iostream>
#include <openssl/evp.h>
#include <openssl/pem.h>
#include <openssl/ec.h>
#include <openssl/err.h>
void handleErrors()
{
unsigned long errCode;
char errBuff[256];
while ((errCode = ERR_get_error()) != 0)
{
ERR_error_string_n(errCode, errBuff, sizeof(errBuff));
std::cerr << "OpenSSL error: " << errBuff << std::endl;
}
throw std::runtime_error("OpenSSL error");
}
EC_KEY *loadPrivateKey(const std::string &privateKeyPath)
{
FILE *fp = fopen(privateKeyPath.c_str(), "rb");
if (fp == nullptr)
{
std::cerr << "Failed to open private key file" << std::endl;
return nullptr;
}
EC_KEY *ecKey = PEM_read_ECPrivateKey(fp, nullptr, nullptr, nullptr);
if (ecKey == nullptr)
{
std::cerr << "Failed to read private key" << std::endl;
fclose(fp);
return nullptr;
}
fclose(fp);
return ecKey;
}
int main()
{
const std::string privateKeyPath = "/path/to/private_key.pem";
OpenSSL_add_all_algorithms();
ERR_load_crypto_strings();
EC_KEY *privateKey = loadPrivateKey(privateKeyPath);
if (privateKey == nullptr)
{
handleErrors();
return 1;
}
// 输出解密后的私钥信息
const BIGNUM *privateKeyBN = EC_KEY_get0_private_key(privateKey);
char *privateKeyHex = BN_bn2hex(privateKeyBN);
std::cout << "Decrypted private key: " << privateKeyHex << std::endl;
OPENSSL_free(privateKeyHex);
EC_KEY_free(privateKey);
EVP_cleanup();
ERR_free_strings();
return 0;
}
```
在示例代码中,我们定义了 `loadPrivateKey` 函数,用于加载包含加密的 SM2 私钥的 PEM 文件。然后,我们使用 `PEM_read_ECPrivateKey` 函数读取并解密私钥。
请确保将 `privateKeyPath` 替换为你实际的私钥文件路径。
编译并运行示例代码,将会输出解密后的 SM2 私钥信息。请注意,你需要在编译时链接 OpenSSL 库,方法请参考前面的回答。
如果你遇到问题或错误,请确保 OpenSSL 库版本正确,并检查私钥文件是否正确。
相关推荐
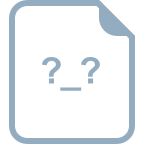
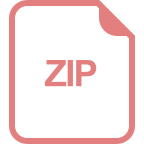
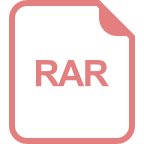












