c语言链表打出实验设备管理系统
时间: 2023-12-21 15:31:03 浏览: 70
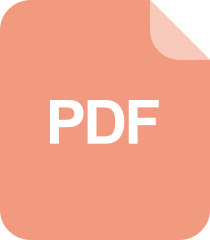
C语言链表实现图书管理系统
以下是C语言链表实现设备管理系统的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
// 设备结构体
typedef struct Device {
char name[20]; // 设备名称
int id; // 设备编号
struct Device *next; // 指向下一个设备的指针
} Device;
// 创建设备链表
Device *createDeviceList() {
Device *head = (Device *)malloc(sizeof(Device)); // 创建头结点
head->next = NULL;
return head;
}
// 添加设备
void addDevice(Device *head, char *name, int id) {
Device *newDevice = (Device *)malloc(sizeof(Device)); // 创建新设备结点
strcpy(newDevice->name, name);
newDevice->id = id;
newDevice->next = NULL;
Device *p = head;
while (p->next != NULL) {
p = p->next;
}
p->next = newDevice;
}
// 删除设备
void deleteDevice(Device *head, int id) {
Device *p = head->next;
Device *pre = head;
while (p != NULL) {
if (p->id == id) {
pre->next = p->next;
free(p);
return;
}
pre = p;
p = p->next;
}
printf("设备编号为%d的设备不存在\n", id);
}
// 修改设备
void modifyDevice(Device *head, int id, char *newName) {
Device *p = head->next;
while (p != NULL) {
if (p->id == id) {
strcpy(p->name, newName);
return;
}
p = p->next;
}
printf("设备编号为%d的设备不存在\n", id);
}
// 查找设备
void searchDevice(Device *head, int id) {
Device *p = head->next;
while (p != NULL) {
if (p->id == id) {
printf("设备编号:%d,设备名称:%s\n", p->id, p->name);
return;
}
p = p->next;
}
printf("设备编号为%d的设备不存在\n", id);
}
// 输出设备列表
void printDeviceList(Device *head) {
Device *p = head->next;
while (p != NULL) {
printf("设备编号:%d,设备名称:%s\n", p->id, p->name);
p = p->next;
}
}
// 清空设备列表
void clearDeviceList(Device *head) {
Device *p = head->next;
while (p != NULL) {
Device *temp = p;
p = p->next;
free(temp);
}
head->next = NULL;
}
int main() {
Device *head = createDeviceList(); // 创建设备链表
// 添加设备
addDevice(head, "设备1", 1);
addDevice(head, "设备2", 2);
addDevice(head, "设备3", 3);
// 输出设备列表
printf("设备列表:\n");
printDeviceList(head);
// 修改设备
modifyDevice(head, 2, "修改后的设备2");
printf("修改后的设备列表:\n");
printDeviceList(head);
// 删除设备
deleteDevice(head, 3);
printf("删除设备后的设备列表:\n");
printDeviceList(head);
// 查找设备
searchDevice(head, 2);
// 清空设备列表
clearDeviceList(head);
printf("清空设备列表后的设备列表:\n"); printDeviceList(head);
return 0;
}
```
阅读全文
相关推荐
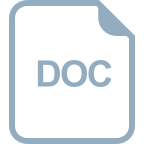
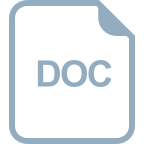
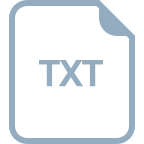
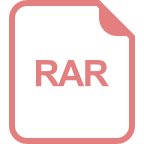
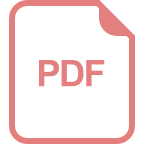
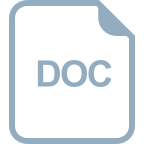
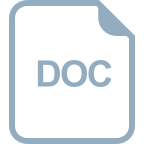
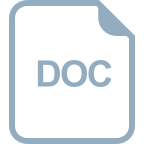


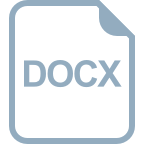
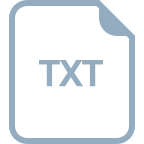
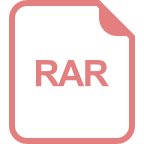
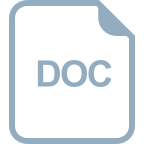
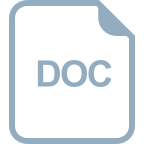


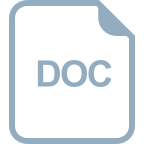
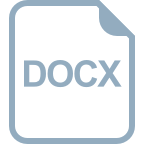