按要求用JAVA语言完成文档中的题目,要求顶点坐标从对应的72度坐标开始输出,随后依次0度288度216度144度的坐标
时间: 2024-10-02 20:03:11 浏览: 21
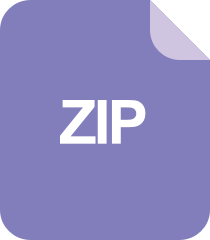
七参数坐标转换Java语言代码
为了完成这个任务,我们需要创建一个Java程序来计算并打印出给定外切圆半径的正五边形的顶点坐标。我们将按照指定的角度顺序输出这些坐标:72度(对应第一个点),然后是0度,288度,216度以及最后的144度。这里我们需要注意的是,角度是以逆时针方向测量,并且是从正x轴开始的。下面给出符合需求的代码示例:
```java
import java.util.Scanner;
public class PentagonCoordinates {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// 提示用户输入外切圆半径
System.out.println("请输入正五边形外切圆的半径:");
double radius = scanner.nextDouble();
// 计算每个顶点的坐标
double angle72 = Math.toRadians(72); // 将角度转换为弧度
double x1 = roundToTwoDecimals(radius * Math.cos(angle72));
double y1 = roundToTwoDecimals(radius * Math.sin(angle72));
double angle0 = Math.toRadians(0);
double x2 = roundToTwoDecimals(radius * Math.cos(angle0));
double y2 = roundToTwoDecimals(radius * Math.sin(angle0));
double angle288 = Math.toRadians(288);
double x3 = roundToTwoDecimals(radius * Math.cos(angle288));
double y3 = roundToTwoDecimals(radius * Math.sin(angle288));
double angle216 = Math.toRadians(216);
double x4 = roundToTwoDecimals(radius * Math.cos(angle216));
double y4 = roundToTwoDecimals(radius * Math.sin(angle216));
double angle144 = Math.toRadians(144);
double x5 = roundToTwoDecimals(radius * Math.cos(angle144));
double y5 = roundToTwoDecimals(radius * Math.sin(angle144));
// 输出结果
System.out.printf("(%.2f, %.2f)\n", x1, y1);
System.out.printf("(%.2f, %.2f)\n", x2, y2);
System.out.printf("(%.2f, %.2f)\n", x3, y3);
System.out.printf("(%.2f, %.2f)\n", x4, y4);
System.out.printf("(%.2f, %.2f)", x5, y5);
scanner.close();
}
private static double roundToTwoDecimals(double value) {
return Math.round(value * 100.0) / 100.0;
}
}
```
在这个例子中,`roundToTwoDecimals()` 方法用于将浮点数四舍五入到小数点后两位。这样可以确保输出格式满足题目要求。此外,使用 `Math.toRadians()` 函数将角度由度转换成弧度制,因为 Java 中的三角函数需要以弧度作为参数。最后,请确保在提交代码前仔细检查所有的输出格式是否与题目描述相匹配。
阅读全文
相关推荐
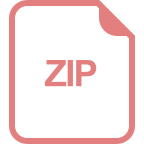
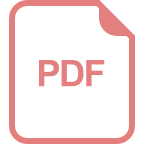


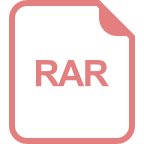
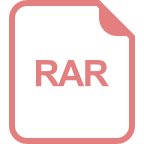
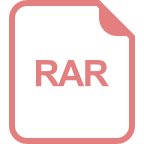
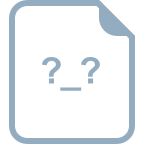
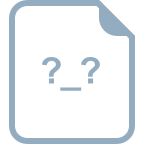
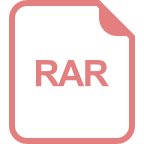
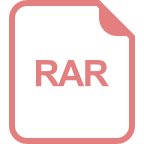
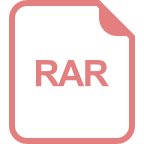