stm32f103c8t6通过usart1接收一个字符串
时间: 2024-05-12 22:12:30 浏览: 27
您好,要实现 STM32F103C8T6 通过 USART1 接收一个字符串,需要进行以下步骤:
1. 配置串口工作模式:初始化 USART1,使其工作在 UART 模式下,设置波特率、数据位、停止位和校验位等参数。
2. 配置串口接收中断:开启 USART1 的接收中断,使其能够在接收到数据时触发中断。
3. 编写中断服务函数:当 USART1 接收到数据时,会触发中断服务函数,在中断服务函数中,我们可以读取接收到的数据,并将其存储在一个缓冲区中。
4. 解析接收到的字符串:当接收到完整的字符串后,我们需要解析该字符串,并进行相应的操作。
下面是具体的代码实现(仅供参考):
```
#include "stm32f10x.h"
#define BUFFER_SIZE 50
uint8_t rx_buffer[BUFFER_SIZE];
uint8_t rx_index = 0;
void USART1_IRQHandler(void)
{
if (USART_GetITStatus(USART1, USART_IT_RXNE) != RESET) {
uint8_t data = USART_ReceiveData(USART1);
if (data == '\n') {
rx_buffer[rx_index] = '\0';
// 当接收到完整的字符串后,在这里添加相应的操作
rx_index = 0;
} else {
rx_buffer[rx_index++] = data;
}
}
}
int main(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
USART_InitTypeDef USART_InitStructure;
NVIC_InitTypeDef NVIC_InitStructure;
// 打开 GPIOA 和 USART1 的时钟
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_USART1, ENABLE);
// 配置 USART1 的引脚
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_9; // TX
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_10; // RX
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IN_FLOATING;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 配置 USART1 的工作参数
USART_InitStructure.USART_BaudRate = 115200;
USART_InitStructure.USART_WordLength = USART_WordLength_8b;
USART_InitStructure.USART_StopBits = USART_StopBits_1;
USART_InitStructure.USART_Parity = USART_Parity_No;
USART_InitStructure.USART_HardwareFlowControl = USART_HardwareFlowControl_None;
USART_InitStructure.USART_Mode = USART_Mode_Rx | USART_Mode_Tx;
USART_Init(USART1, &USART_InitStructure);
// 配置 USART1 的接收中断
USART_ITConfig(USART1, USART_IT_RXNE, ENABLE);
// 配置 NVIC 中断优先级和使能 USART1 的中断
NVIC_InitStructure.NVIC_IRQChannel = USART1_IRQn;
NVIC_InitStructure.NVIC_IRQChannelPreemptionPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelSubPriority = 0;
NVIC_InitStructure.NVIC_IRQChannelCmd = ENABLE;
NVIC_Init(&NVIC_InitStructure);
// 启动 USART1
USART_Cmd(USART1, ENABLE);
while (1) {
// 在这里添加其他操作
}
}
```
相关推荐
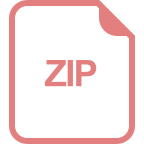
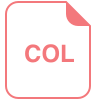
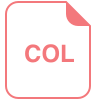
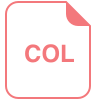
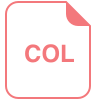
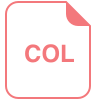









