python计算每年每月的最后一天
时间: 2024-09-15 15:04:24 浏览: 87
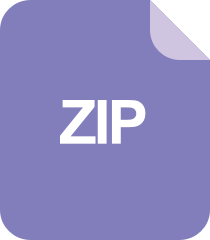
python项目 —— 圣诞节倒计时
在Python中,你可以使用`calendar`模块来计算每个月的最后一天。`calendar`模块是Python的标准库之一,提供了处理日期和时间的实用功能。以下是一个使用`calendar`模块计算每个月最后一天的示例代码:
```python
import calendar
# 指定年份和月份
year = 2023
month = 4 # 4代表4月
# 创建一个文本日历实例
cal = calendar.TextCalendar(calendar.SUNDAY)
# 获取指定年月的日历字符串
month_calendar_str = cal.formatmonth(year, month)
# 将字符串分割成行
lines = month_calendar_str.splitlines()
# 遍历每一行,找到最后一行即为月末
for line in lines:
if line.strip():
last_day = line.split()[-1]
break
# 输出月末
print(f"{year}年{month}月的最后一天是:{last_day}")
```
这段代码首先导入了`calendar`模块,并指定了要查询的年份和月份。然后,它创建了一个`TextCalendar`实例,该实例默认使用星期天作为一周的第一天。接着,代码使用`formatmonth`方法得到了指定年月的日历字符串,并将其分割成单独的行。通过遍历这些行,找到最后一行,该行中的最后一个元素即为该月的最后一天。
阅读全文
相关推荐
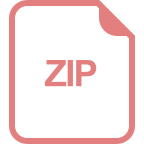
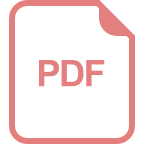
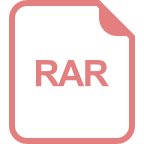















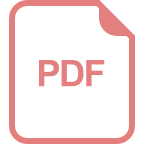