pthread_create功能
时间: 2023-11-17 20:06:03 浏览: 25
pthread_create()函数是POSIX线程库中的一个函数,用于创建一个新的线程。它接受四个参数:指向线程标识符的指针、线程属性、指向函数的指针和传递给该函数的参数。该函数返回0表示成功,否则返回错误代码。
以下是一个简单的pthread_create()函数的例子:
```c
#include <pthread.h>
#include <stdio.h>
void *myThreadFun(void *vargp)
{
sleep(1);
printf("Printing from Thread \n");
return NULL;
}
int main()
{
pthread_t thread_id;
printf("Before Thread\n");
pthread_create(&thread_id, NULL, myThreadFun, NULL);
pthread_join(thread_id, NULL);
printf("After Thread\n");
exit(0);
}
```
相关问题
pthread_create源码
抱歉,我无法直接提供代码。但是我可以给你一个关于`pthread_create`函数的概览和使用方法。
`pthread_create`是Linux下用于创建新线程的函数,其原型如下:
```c
#include <pthread.h>
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine) (void *), void *arg);
```
函数参数解释如下:
- `thread`:指向`pthread_t`类型的指针,用于存储新线程的标识符。
- `attr`:指向`pthread_attr_t`类型的指针,用于设置新线程的属性(可为NULL,表示使用默认属性)。
- `start_routine`:新线程的起始函数,它是一个函数指针,接受一个`void*`类型的参数,并返回一个`void*`类型的值。
- `arg`:传递给起始函数的参数。
`pthread_create`函数的功能是创建一个新线程,该线程会调用`start_routine`函数,并将`arg`作为参数传递给它。新线程创建成功返回0,失败返回非零错误码。
需要注意的是,线程函数的定义必须符合要求:接受一个`void*`类型的参数并返回一个`void*`类型的值。如果需要传递多个参数,可以使用结构体或者指针来进行传递。
希望这能帮到你!如果有更多问题,请随时提问。
pthread_create()
### 回答1:
pthread_create() 是一个 POSIX 线程库函数,用于创建一个新的线程。它接受四个参数:指向线程标识符的指针、线程属性、指向函数的指针和传递给函数的参数。它返回一个整数,表示线程创建是否成功。
### 回答2:
pthread_create()是一个用于创建一个新线程的函数。它的语法如下:
int pthread_create(pthread_t *thread, const pthread_attr_t *attr,
void *(*start_routine) (void *), void *arg);
其中,thread是指向线程标识符的指针,attr是指向线程属性的指针,start_routine是指向线程函数的指针,arg是传递给线程函数的参数。
pthread_create()函数的作用是创建一个新线程,并将其添加到调用线程之后的线程序列中。新线程从start_routine函数开始执行,并且传递arg作为参数。
pthread_create()函数在成功创建新线程时返回0,否则返回错误代码。通过检查返回值,可以确定线程是否成功创建。
创建新线程时,可以通过attr参数传递线程属性,例如设置线程的分离状态、设置线程的堆栈大小等。
线程函数start_routine的原型是void *(*start_routine) (void *),其返回类型为void指针,参数为一个void指针。线程函数的功能由程序员自行定义,可以实现任意需要在新线程中执行的操作。
新线程的执行是并发的,它将与调用线程同时执行,不会等待调用pthread_create()函数的返回。因此,在创建新线程后,需要使用pthread_join()函数等待新线程的终止,以防止主线程先于新线程运行结束。
总结来说,pthread_create()函数是一个用于创建新线程的函数,通过该函数可以创建并发执行的线程,并执行指定的线程函数。通过传递参数,可以向线程函数传递需要的数据。
### 回答3:
pthread_create() 是 POSIX 线程库中的一个函数,用于创建一个新的线程。其函数原型如下:
```c
int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine)(void*), void *arg);
```
参数解释:
- thread:指向线程 ID 的指针,用于接收新创建的线程的标识符;
- attr:新线程的属性,使用默认属性时可以设置为 NULL;
- start_routine:新线程的入口函数,它是一个指向函数的指针,该函数接收一个 void* 类型参数并返回一个 void* 类型的结果;
- arg:传递给新线程入口函数的参数,可以是任意类型的指针。
pthread_create() 函数的作用是创建一个新的线程,并将新线程的相关信息保存到 thread 指向的位置中。新线程的属性可以通过 attr 参数进行配置,例如设置线程的优先级、栈大小等。新线程的入口函数通过 start_routine 参数指定,传入的参数通过 arg 参数传递。
pthread_create() 函数成功创建新线程时返回 0,失败时返回一个非零的错误码。在创建线程成功后,可以使用 pthread_join() 函数等待新线程的结束,并获取新线程的返回值。
使用 pthread_create() 函数可以实现多线程并发执行,提高程序的处理能力和效率。多线程编程可以充分利用现代计算机多核心的优势,将任务拆分为多个线程并行处理,从而提高程序的响应速度和吞吐量。同时,多线程编程也需要注意线程同步和资源竞争等问题,以确保数据的一致性和正确性。
相关推荐
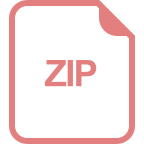
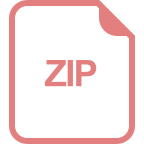
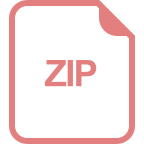













