利用XGBOOST建立回归模型,并利用gridsearchcv寻找最优参数,给出代码
时间: 2024-11-05 07:33:24 浏览: 14
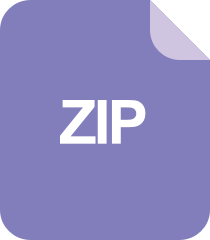
XGBoost算法Python实战(代码).zip

XGBoost是一个流行的梯度提升库,用于分类和回归任务。下面是一个简单的例子,展示如何使用XGBoost建立回归模型,并使用GridSearchCV寻找最优参数:
```python
import xgboost as xgb
from sklearn.model_selection import GridSearchCV
from sklearn.datasets import load_boston
from sklearn.preprocessing import StandardScaler
from sklearn.metrics import mean_squared_error
# 加载波士顿房价数据集
boston = load_boston()
X, y = boston.data, boston.target
# 数据预处理
scaler = StandardScaler()
X_scaled = scaler.fit_transform(X)
# 定义XGBoost模型并设置参数网格
param_grid = {
'n_estimators': [100, 200, 300],
'learning_rate': [0.1, 0.05, 0.01],
'max_depth': [3, 5, 7],
'gamma': [0.0, 0.1, 0.2]
}
# 初始化XGBoost模型
model = xgb.XGBRegressor()
# 使用GridSearchCV寻找最优参数
grid_search = GridSearchCV(model, param_grid, scoring='neg_mean_squared_error', cv=5, n_jobs=-1)
grid_search.fit(X_scaled, y)
# 获取最佳参数
best_params = grid_search.best_params_
print(f"Best parameters found: {best_params}")
# 使用最佳参数训练最终模型
best_xgb_reg = grid_search.best_estimator_
best_xgb_reg.fit(X_scaled, y)
# 预测测试集
X_test, _ = boston.data[-10:], boston.target[-10:]
test_X_scaled = scaler.transform(X_test)
y_pred = best_xgb_reg.predict(test_X_scaled)
# 计算评估指标
mse = mean_squared_error(y_test, y_pred)
print("均方误差 (MSE):", mse)
```
在这个例子中,我们首先加载数据,标准化特征,然后创建一个XGBoost回归器,定义了一个参数网格。接着使用`GridSearchCV`搜索最优参数,最后使用找到的最佳参数训练模型并对测试集做预测。
阅读全文
相关推荐
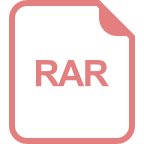
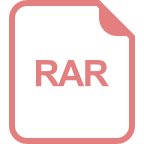











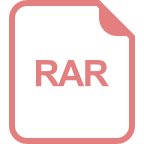
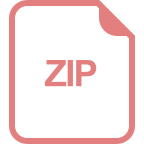
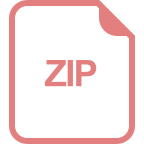
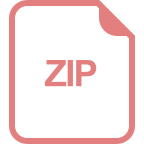
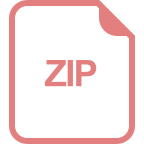