创建 DataFrame 对象students记录3名学生的信息,行索引为数字序号,列索引为age、weight和height。python
时间: 2024-11-13 15:41:50 浏览: 16
在Python的pandas库中,你可以创建DataFrame对象来存储结构化的数据。以下是如何创建一个名为`students`的DataFrame,包含3名学生的年龄(age)、体重(weight)和身高(height)信息:
```python
import pandas as pd
# 定义字典来表示每个学生的数据
data = {
'age': [18, 20, 22],
'weight': [65, 70, 75],
'height': [170, 175, 180]
}
# 使用字典创建DataFrame
students = pd.DataFrame(data)
# 设置行索引为数字序号(默认)
students.index = range(1, len(students)+1)
# 输出 DataFrame 的前几行
print(students.head())
```
在这个例子中,`range(1, len(students)+1)`设置了从1开始到3(总共有3名学生)的整数作为行索引。运行上述代码后,你会得到一个显示3名学生基本信息的DataFrame。
相关问题
从字典对象创建DataFrame对象,索引为labels age NaN
可以使用 pandas 库中的 DataFrame() 函数从字典对象创建 DataFrame 对象,并指定索引为 labels,其中 age 列的值为 NaN。示例代码如下:
```python
import pandas as pd
# 定义字典对象
data = {'name': ['Alice', 'Bob', 'Charlie', 'David'],
'age': [30, 25, 20, None],
'gender': ['F', 'M', 'M', 'M']}
# 创建 DataFrame 对象
df = pd.DataFrame(data, index=['A', 'B', 'C', 'D'], columns=['name', 'age', 'gender'])
# 打印 DataFrame 对象
print(df)
```
输出结果如下:
```
name age gender
A Alice 30.0 F
B Bob 25.0 M
C Charlie 20.0 M
D David NaN M
```
其中,data 是包含数据的字典对象,index 参数指定索引为 labels,columns 参数指定 DataFrame 对象的列名。在创建 DataFrame 对象时,age 列的值为 None,表示缺失值,因此在输出结果中显示为 NaN。
根据数组创建dataframe行索引为one two,列索引为col1
可以使用 pandas.DataFrame 函数来创建一个空的 DataFrame,然后使用 reindex 函数来设置行索引和列索引:
```python
import pandas as pd
# 创建一个空的 DataFrame
df = pd.DataFrame()
# 设置行索引为 "one" 和 "two"
df = df.reindex(["one", "two"])
# 设置列索引为 "col1"
df["col1"] = None
# 输出结果
print(df)
```
输出结果:
```
col1
one None
two None
```
注意,这里我们首先创建了一个空的 DataFrame,然后使用 reindex 函数来设置行索引,最后再添加一个列索引。如果直接在 DataFrame 函数中指定行索引和列索引,也可以达到同样的效果:
```python
import pandas as pd
# 直接指定行索引和列索引
df = pd.DataFrame(index=["one", "two"], columns=["col1"])
# 输出结果
print(df)
```
输出结果:
```
col1
one NaN
two NaN
```
阅读全文
相关推荐
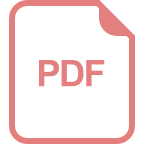
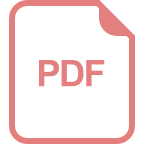














