Python五子棋程序
时间: 2023-12-25 17:27:52 浏览: 113
以下是一个简单的Python五子棋程序的实现,使用Pygame库进行图形界面的绘制和交互:
```python
import pygame
# 初始化Pygame
pygame.init()
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
BLUE = (0, 0, 255)
GREEN = (0, 255, 0)
# 设置棋盘大小和格子大小
BOARD_SIZE = 600
GRID_SIZE = 30
# 创建棋盘
board = [[0] * 20 for i in range(20)]
# 创建屏幕
screen = pygame.display.set_mode((BOARD_SIZE, BOARD_SIZE))
# 设置窗口标题
pygame.display.set_caption("五子棋")
# 绘制棋盘
def draw_board():
for i in range(20):
pygame.draw.line(screen, BLACK, [GRID_SIZE, (i+1)*GRID_SIZE], [BOARD_SIZE-GRID_SIZE, (i+1)*GRID_SIZE], 2)
pygame.draw.line(screen, BLACK, [(i+1)*GRID_SIZE, GRID_SIZE], [(i+1)*GRID_SIZE, BOARD_SIZE-GRID_SIZE], 2)
# 绘制棋子
def draw_piece(row, col, player):
if player == 1:
color = BLACK
else:
color = WHITE
pygame.draw.circle(screen, color, [(col+1)*GRID_SIZE, (row+1)*GRID_SIZE], GRID_SIZE//2-2)
# 判断胜负
def check_win(row, col, player):
count = 0
# 横向
for i in range(max(0, col-4), min(20, col+5)):
if board[row][i] == player:
count += 1
if count == 5:
return True
else:
count = 0
# 纵向
count = 0
for i in range(max(0, row-4), min(20, row+5)):
if board[i][col] == player:
count += 1
if count == 5:
return True
else:
count = 0
# 左上到右下
count = 0
for i in range(-4, 5):
r = row + i
c = col + i
if r < 0 or r >= 20 or c < 0 or c >= 20:
continue
if board[r][c] == player:
count += 1
if count == 5:
return True
else:
count = 0
# 左下到右上
count = 0
for i in range(-4, 5):
r = row - i
c = col + i
if r < 0 or r >= 20 or c < 0 or c >= 20:
continue
if board[r][c] == player:
count += 1
if count == 5:
return True
else:
count = 0
return False
# 游戏主循环
def main():
# 初始化游戏
player = 1
game_over = False
# 游戏循环
while not game_over:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
elif event.type == pygame.MOUSEBUTTONDOWN:
if player == 1:
row = int(round((event.pos[1]-GRID_SIZE)/GRID_SIZE))
col = int(round((event.pos[0]-GRID_SIZE)/GRID_SIZE))
if row >= 0 and row < 20 and col >= 0 and col < 20 and board[row][col] == 0:
draw_piece(row, col, player)
board[row][col] = player
if check_win(row, col, player):
print("Player 1 wins!")
game_over = True
player = 2
else:
row = int(round((event.pos[1]-GRID_SIZE)/GRID_SIZE))
col = int(round((event.pos[0]-GRID_SIZE)/GRID_SIZE))
if row >= 0 and row < 20 and col >= 0 and col < 20 and board[row][col] == 0:
draw_piece(row, col, player)
board[row][col] = player
if check_win(row, col, player):
print("Player 2 wins!")
game_over = True
player = 1
# 绘制棋盘
screen.fill(WHITE)
draw_board()
for i in range(20):
for j in range(20):
if board[i][j] == 1:
draw_piece(i, j, 1)
elif board[i][j] == 2:
draw_piece(i, j, 2)
# 更新屏幕
pygame.display.flip()
# 退出Pygame
pygame.quit()
if __name__ == '__main__':
main()
```
阅读全文
相关推荐
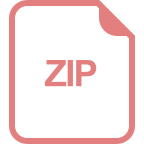
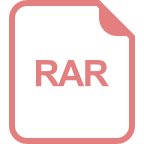
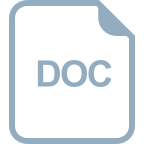
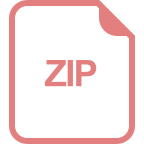
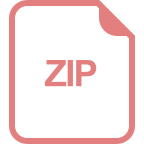
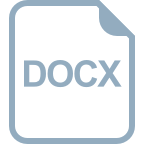
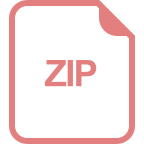
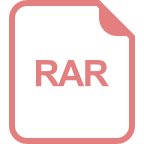
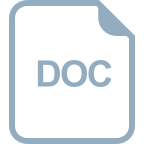

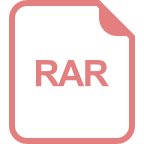
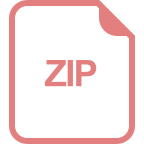