使用idea,在springBoot中定义一个yml配置文件,描述员工的基本信息,包括姓名(字符串)、出生年月(日期)、兴趣爱好(列表)、性别(字符串)等信息。请用上述yml配置文件中的的员工属性值,定义员工类,选择合适的方法实现配置文件属性值的注入。使用@Value
时间: 2024-09-24 14:12:30 浏览: 32
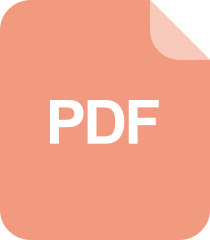
springboot 在xml里读取yml的配置信息的示例代码

在IntelliJ IDEA中,为了在Spring Boot项目中定义一个YAML配置文件来存储员工信息,首先你需要创建一个新的YAML文件,例如`employee.yml`,内容如下:
```yaml
employees:
- id: 001
name: 张三
birthDate: 1990-01-01
hobbies:
- 阅读
- 游泳
gender: 男
- id: 002
name: 李四
birthDate: 1995-06-15
hobbies:
- 篮球
- 跑步
gender: 女
```
然后在Spring Boot中,我们需要创建一个Employee实体类,并使用`@ConfigurationProperties`注解将YAML文件中的数据映射到这个类上:
```java
import org.springframework.boot.context.properties.ConfigurationProperties;
import java.time.LocalDate;
import java.util.List;
@ConfigurationProperties(prefix = "employees")
public class Employee {
private String id;
private String name;
private LocalDate birthDate;
private List<String> hobbies;
private String gender;
// Getters and Setters for each property
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public LocalDate getBirthDate() {
return birthDate;
}
public void setBirthDate(LocalDate birthDate) {
this.birthDate = birthDate;
}
public List<String> getHobbies() {
return hobbies;
}
public void setHobbies(List<String> hobbies) {
this.hobbies = hobbies;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
}
```
现在,Spring Boot会自动将`employee.yml`中的配置项注入到`Employee`类对应的属性中。在需要的地方,你只需要通过@Autowired或者@Bean注解来注入Employee bean即可,Spring会自动处理属性的绑定:
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.stereotype.Component;
@Component
public class AppConfig implements CommandLineRunner {
@Autowired
private Employee employee;
@Override
public void run(String... args) throws Exception {
System.out.println("Employee Name: " + employee.getName());
// 其他属性的访问也类似
}
}
```
在这个例子中,我们使用了`@Value`注解,但在Spring Boot的`@ConfigurationProperties`中,它通常是隐式使用的,不需要显式声明。`@Value`通常用于单个值的配置,而`@ConfigurationProperties`更适用于复杂的数据结构映射。
阅读全文
相关推荐
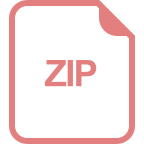
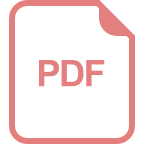














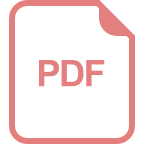
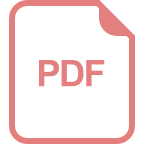