C++ AES CBC 加密 代码 使用 openssl
时间: 2024-12-01 16:20:36 浏览: 13
在C++中使用OpenSSL库进行AES加密,特别是CBC模式,你需要包括相关的头文件并初始化OpenSSL库。以下是一个简单的示例,展示了如何对数据进行AES-CBC加密和解密:
```cpp
#include <openssl/aes.h>
#include <openssl/evp.h>
#include <string>
std::string encrypt_CBC(const std::string& plaintext, const std::string& key, const std::string& iv) {
AES_KEY aes_key;
unsigned char iv_bytes[AES_BLOCK_SIZE];
memcpy(iv_bytes, iv.c_str(), AES_BLOCK_SIZE);
// Initialize the AES key
if (AES_set_encrypt_key((unsigned char*)key.c_str(), key.length() * 8, &aes_key) != AES_SUCCESS) {
throw std::runtime_error("AES key initialization failed");
}
// Create a buffer for the ciphertext and initialize it with the IV
size_t ciphertext_len = plaintext.length() + AES_BLOCK_SIZE;
unsigned char* ciphertext = new unsigned char[ciphertext_len];
memcpy(ciphertext, iv_bytes, AES_BLOCK_SIZE); // Add the IV at the beginning
// Encrypt the plaintext in CBC mode
size_t blocks = plaintext.length() / AES_BLOCK_SIZE;
for (size_t i = 0; i < blocks; ++i) {
AES_cbc_encrypt(reinterpret_cast<const unsigned char*>(plaintext.data() + i * AES_BLOCK_SIZE), ciphertext + i * AES_BLOCK_SIZE, AES_BLOCK_SIZE, &aes_key, iv_bytes, AES_ENCRYPT);
}
if (plaintext.length() % AES_BLOCK_SIZE > 0) { // Padding for last block
AES_cbc_encrypt(reinterpret_cast<const unsigned char*>(plaintext.data() + blocks * AES_BLOCK_SIZE), ciphertext + blocks * AES_BLOCK_SIZE, plaintext.length() % AES_BLOCK_SIZE, &aes_key, iv_bytes, AES_ENCRYPT);
}
std::string encrypted_text(reinterpret_cast<char*>(ciphertext), ciphertext_len - AES_BLOCK_SIZE);
delete[] ciphertext;
return encrypted_text;
}
std::string decrypt_CBC(const std::string& ciphertext, const std::string& key, const std::string& iv) {
// Similar to encrypt function, but use AES_decrypt instead of AES_encrypt
// Remember to properly handle padding when decrypting
// ...
}
int main() {
try {
std::string plaintext = "This is a secret message";
std::string key = "mysecretkey1234567890123456"; // 256-bit key
std::string iv = "0123456789abcdef01234567"; // 16-byte initialization vector
std::string encrypted = encrypt_CBC(plaintext, key, iv);
std::string decrypted = decrypt_CBC(encrypted, key, iv);
if (decrypted == plaintext) {
std::cout << "Encryption and decryption successful!" << std::endl;
} else {
std::cerr << "Decryption failed!" << std::endl;
}
} catch (const std::exception& e) {
std::cerr << "Error: " << e.what() << std::endl;
}
return 0;
}
```
请注意,上述代码仅作为一个基本示例,并未包含完整的错误处理和padding处理。实际应用中,你需要确保IV的安全性和正确的填充。在`decrypt_CBC`函数中,你需要根据OpenSSL文档调整处理方式。
阅读全文
相关推荐
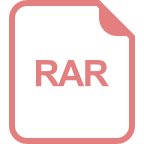
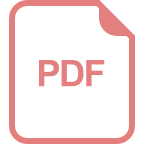
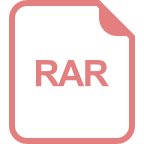
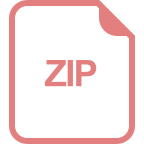
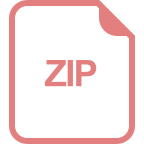
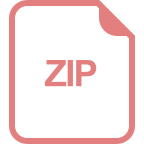
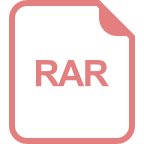
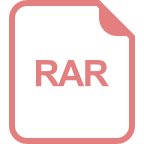
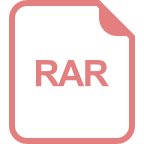
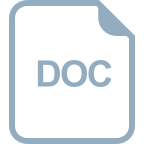
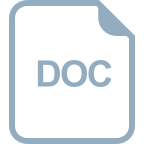


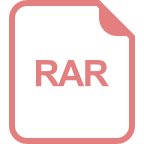
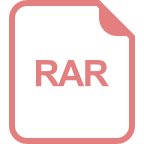
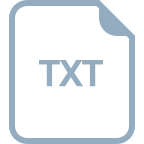
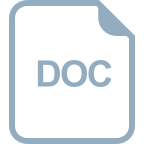
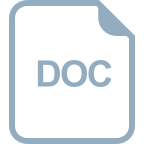
