python heappush
时间: 2023-08-28 19:07:01 浏览: 54
heappush is a function in the Python heapq module. It is used to push an element into a heap while preserving the heap property. The heap can be either a list or any other data structure that supports heap operations.
Here is an example of how to use heappush in Python:
```python
import heapq
heap = []
heapq.heappush(heap, 10)
heapq.heappush(heap, 5)
heapq.heappush(heap, 15)
print(heap) # Output: [5, 10, 15]
```
In this example, we create an empty heap using a list. Then, we use heappush to insert elements into the heap. The resulting heap maintains the smallest element at the root.
相关问题
python 最小堆
Python中的最小堆是一种数据结构,它是一种特殊的二叉树,其中每个节点的值都小于或等于其子节点的值。最小堆通常用于实现优先级队列和排序算法。
在Python中,可以使用heapq模块来实现最小堆。heapq模块提供了一些函数来操作堆,包括插入元素、删除最小元素等操作。
下面是使用heapq模块实现最小堆的示例代码:
```python
import heapq
# 创建一个空的最小堆
heap = []
# 插入元素到最小堆
heapq.heappush(heap, 5)
heapq.heappush(heap, 3)
heapq.heappush(heap, 8)
heapq.heappush(heap, 1)
# 获取最小元素
min_element = heapq.heappop(heap)
print(min_element) # 输出:1
# 获取最小元素(不删除)
min_element = heap[0]
print(min_element) # 输出:3
```
上述代码首先创建了一个空的最小堆,然后使用`heappush`函数向堆中插入元素。`heappop`函数用于删除并返回最小元素,而不删除最小元素可以直接通过索引访问堆的第一个元素。
P1030python
根据提供的引用内容,P1030是一道题目,需要使用Python编写程序来解决。具体来说,这道题目要求我们实现一个字符串的哈夫曼编码,即将给定的字符串转换为哈夫曼编码后输出。其中,哈夫曼编码是一种可变长度编码,通过将出现频率较高的字符用较短的编码表示,从而达到压缩数据的目的。
以下是一个Python实现哈夫曼编码的例子:
```python
from heapq import heappush, heappop, heapify
from collections import defaultdict
def huffman_encoding(s):
# 统计字符出现的频率
freq = defaultdict(int)
for c in s:
freq[c] += 1
# 构建哈夫曼树
heap = [[wt, [sym, ""]] for sym, wt in freq.items()]
heapify(heap)
while len(heap) > 1:
lo = heappop(heap)
hi = heappop(heap)
for pair in lo[1:]:
pair[1] = '0' + pair[1]
for pair in hi[1:]:
pair[1] = '1' + pair[1]
heappush(heap, [lo[0] + hi[0]] + lo[1:] + hi[1:])
# 构建编码表
code = dict(heappop(heap)[1:])
return code
s = input()
code = huffman_encoding(s)
for c in s:
print(code[c], end="")
```
相关推荐
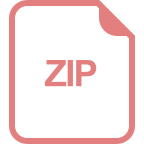
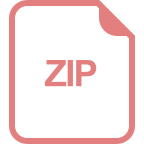












