基类Shape类是一个表示形状的抽象类,Area( )为求图形面积的方法。请从Shape类派生出三角形类(Triangle)、圆类(Circle),并实现具体的求面积方法Area( ),并在主函数中多态的实现调用。c#
时间: 2024-10-08 13:03:54 浏览: 31
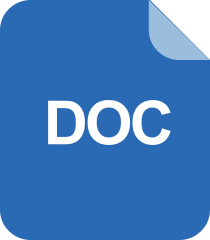
编写一个抽象类Shape

在C#中,你可以这样设计:
首先,我们创建一个抽象基类`Shape`,声明一个虚方法`CalculateArea()`来计算面积:
```csharp
public abstract class Shape
{
public abstract double CalculateArea();
}
```
然后,分别派生两个子类`Triangle`和`Circle`,实现各自的`CalculateArea()`方法:
```csharp
public class Triangle : Shape
{
private double baseLength;
private double height;
public Triangle(double baseLength, double height)
{
this.baseLength = baseLength;
this.height = height;
}
public override double CalculateArea()
{
return 0.5 * baseLength * height;
}
}
public class Circle : Shape
{
private double radius;
public Circle(double radius)
{
this.radius = radius;
}
public override double CalculateArea()
{
return Math.PI * Math.Pow(radius, 2);
}
}
```
最后,在主函数中,我们可以创建`Shape`类型的集合,并通过多态调用`CalculateArea()`方法:
```csharp
using System;
using System.Collections.Generic;
class Program
{
static void Main(string[] args)
{
List<Shape> shapes = new List<Shape>();
shapes.Add(new Triangle(3, 4));
shapes.Add(new Circle(5));
foreach (var shape in shapes)
{
Console.WriteLine($"The area of the shape is: {shape.CalculateArea()}");
}
阅读全文
相关推荐
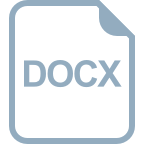











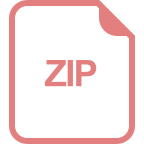
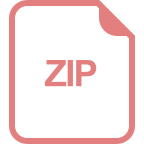
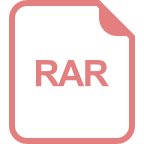
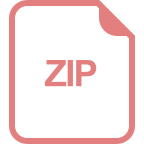
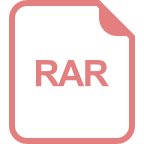