std::condition_variable wait_for
时间: 2023-08-22 19:56:14 浏览: 165
std::condition_variable::wait_for 是一个等待一段时间的函数。它会阻塞当前线程,直到指定的时间段过去或者被通知唤醒。
使用方法如下:
1. 首先,创建一个 std::condition_variable 对象和一个 std::mutex 对象,用于线程间的同步。
2. 然后,在需要等待的线程中调用 wait_for 函数,并传入一个 std::unique_lock<std::mutex> 对象和一个时间段作为参数。std::unique_lock<std::mutex> 对象用于锁定互斥量,时间段用于指定等待的时间。
3. 当等待时间到达或者被其他线程调用 notify_one 或 notify_all 函数通知唤醒时,等待线程会被唤醒并继续执行。
示例代码如下:
```cpp
#include <iostream>
#include <condition_variable>
#include <mutex>
#include <chrono>
std::condition_variable cv;
std::mutex mtx;
void waiting_thread()
{
std::unique_lock<std::mutex> lock(mtx);
// 等待 5 秒钟
if (cv.wait_for(lock, std::chrono::seconds(5)) == std::cv_status::timeout)
{
std::cout << "等待超时!" << std::endl;
}
else
{
std::cout << "等待完成!" << std::endl;
}
}
int main()
{
std::thread t(waiting_thread);
// 主线程休眠 3 秒钟
std::this_thread::sleep_for(std::chrono::seconds(3));
// 唤醒等待线程
cv.notify_one();
t.join();
return 0;
}
```
在上面的示例中,等待线程会等待 5 秒钟,但是在主线程休眠 3 秒钟后唤醒了等待线程,所以等待线程会在等待超时之前被唤醒。
阅读全文
相关推荐
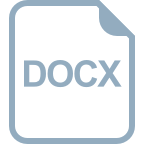
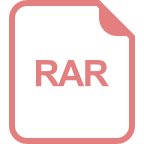
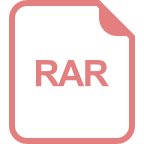















