c++利用头插法或尾插法建立单链表
时间: 2024-09-24 20:14:43 浏览: 39
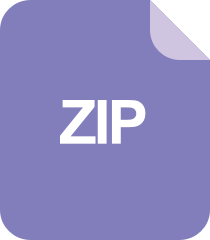
c++头插法和尾插法创建、删除、遍历单链表.zip
在C++中,头插法(Insertion at the beginning)和尾插法(Insertion at the end)是创建单链表常用的方法。这里分别给出这两个操作的基本实现:
1. **头插法**(Insertion at the beginning):
```cpp
class Node {
public:
int data;
Node* next;
// 构造函数
Node(int value) : data(value), next(nullptr) {}
};
class LinkedList {
private:
Node* head;
public:
// 插入新元素到头部
void insertAtBegin(int value) {
Node* newNode = new Node(value);
newNode->next = head; // 新节点指向当前头
head = newNode; // 更新头指针
}
// 其他链表操作...
};
```
在这个例子中,`insertAtBegin`方法首先创建一个新的节点,然后将它的`next`指针指向当前的头节点,最后更新头指针。
2. **尾插法**(Insertion at the end):
```cpp
void insertAtEnd(int value) {
if (head == nullptr) {
head = new Node(value); // 如果链表为空,直接插入
} else {
Node* temp = head;
while (temp->next != nullptr) { // 遍历找到末尾
temp = temp->next;
}
temp->next = new Node(value); // 在最后一个节点之后插入新节点
}
}
```
这个`insertAtEnd`方法检查链表是否为空,如果空则直接在头插入。否则,它通过一个循环找到链表的末尾,并在其之后插入新的节点。
阅读全文
相关推荐
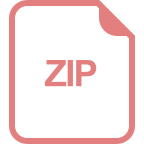
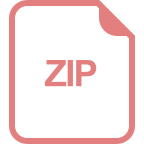

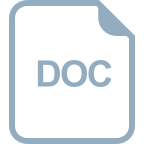
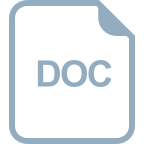











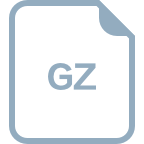
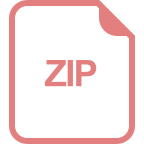