那如果我的 person构造函数 Person(string name, int age, char sex) { _name=name; _age=age; _sex=sex; }这么去写,student构造函数怎么写呢
时间: 2024-12-13 10:18:03 浏览: 9
如果你的`Person`类构造函数已经如你所描述那样简单,那么`Student`类的构造函数可以直接基于这个基础,只增加`uid`这一额外属性。下面是`Student`类的构造函数示例:
```cpp
class Person {
public:
Person(const std::string& name, int age, char sex) : name_(name), age_(age), sex_(sex) {}
private:
std::string name_;
int age_;
char sex_;
};
class Student : public Person { // 继承Person
public:
Student(const std::string& name, int age, char sex, int uid) : Person(name, age, sex), _uid(uid) {
// 如果需要在初始化`Person`属性后再做其他操作,可以在这里添加
}
private:
int _uid;
};
```
在`Student`的构造函数中,首先调用`Person`的构造函数来设置共享的属性,然后设置`_uid`。如果不需要对`_uid`做特别处理,这通常是够用了。
相关问题
Student( string name, int age, char sex,int uid) : Person(name, age, sex), _uid(uid){};把Person(name, age, sex), _uid(uid)放在{}里怎么去编程呢
在这个代码片段中,`Student(string name, int age, char sex, int uid): Person(name, age, sex), _uid(uid)` 是C++的构造函数初始化列表,用于指定`Student`类如何从其基类`Person`派生,并设置私有数据成员`_uid`。这是一种简洁的方式来初始化新创建的`Student`对象的属性。
当你像这样编写:
```cpp
Student s("张三", 20, 'M', 1234);
```
实际上是通过`Student`类的构造函数,隐式地调用了`Person`的构造函数来传递`name`, `age`, 和 `sex`,然后直接设置了`_uid`的值。这里的`{}`并不是在编程上下文中使用的,而是指代整个初始化过程。如果你需要详细解释这部分的语法结构,这里是一个简化版的示例:
```cpp
class Person {
public:
Person(const std::string& name, int age, char sex) : name_(name), age_(age), sex_(sex) {}
private:
std::string name_;
int age_;
char sex_;
};
class Student : public Person { // 继承Person
public:
Student(const std::string& name, int age, char sex, int uid) : Person(name, age, sex), _uid(uid) {} // 初始化列表
private:
int _uid;
};
```
class Person {public: Person(char name[]="XXX", char sex='M',int age=18) { strcpy(this->name,name); this->sex =sex; this->age = age; } virtual void input() { cout << "Enter name:"; cin>> name; cout << "Enter sex:"; cin >> sex; cout << "Enter age:"; cin >> age; } virtual void output() { cout << "name:"<<name << "\t"; cout << "sex:"<< sex << "\t"; cout << "age:"<< sex << age <<endl; } protected: char name[21]; char sex; int age; }; 由Person类派生出如下的派生类Student: class Student:public Person {public: Student(char name[]="XXX", char sex='M',int age=18,char num[]="000000",char class_name[]="XXXXXX"); void input(); void output(); private: char num[10]; //学号 char class_name[21];//班级 }; void writeFile(string filename,Student stud[],int); void readFile(string filename,Student stud[],int); 要求: (1)写出Student类的构造函数、input函数、output函数的类外定义。 (2)写出把一组Student类对象写入文件的writeFile函数的函数体。 (3)写出从文件中读取所有Student类对象的readFile函数的函数体。
(1) Student类的构造函数、input函数、output函数的类外定义如下:
```
Student::Student(char name[], char sex, int age, char num[], char class_name[]) : Person(name, sex, age) {
strcpy(this->num, num);
strcpy(this->class_name, class_name);
}
void Student::input() {
Person::input();
cout << "Enter student number:";
cin >> num;
cout << "Enter class name:";
cin >> class_name;
}
void Student::output() {
Person::output();
cout << "student number:" << num << "\t";
cout << "class name:" << class_name << endl;
}
```
(2) 把一组Student类对象写入文件的writeFile函数的函数体如下:
```
void writeFile(string filename, Student stud[], int n) {
ofstream out(filename, ios::out | ios::binary);
if (!out) {
cout << "Cannot open file!" << endl;
return;
}
out.write(reinterpret_cast<char*>(&n), sizeof(int));
for (int i = 0; i < n; i++) {
out.write(reinterpret_cast<char*>(&stud[i]), sizeof(Student));
}
out.close();
}
```
(3) 从文件中读取所有Student类对象的readFile函数的函数体如下:
```
void readFile(string filename, Student stud[], int n) {
ifstream in(filename, ios::in | ios::binary);
if (!in) {
cout << "Cannot open file!" << endl;
return;
}
int count;
in.read(reinterpret_cast<char*>(&count), sizeof(int));
if (count != n) {
cout << "The number of records in the file does not match the input!" << endl;
in.close();
return;
}
for (int i = 0; i < n; i++) {
in.read(reinterpret_cast<char*>(&stud[i]), sizeof(Student));
}
in.close();
}
```
阅读全文
相关推荐
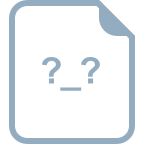
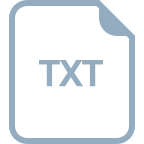
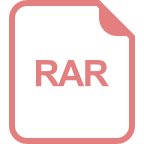













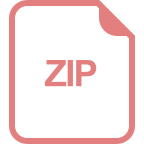