C# winform使用 OxyPlot 每秒钟5000个数据点绘制瀑布图 简单示例代码
时间: 2024-10-22 15:09:46 浏览: 49
在C# WinForms应用程序中使用OxyPlot绘制瀑布图,并每秒更新5000个数据点,可以按照以下步骤进行。首先,确保你已经安装了OxyPlot和OxyPlot.WinForms NuGet包。
以下是一个简单的示例代码,展示如何实现这一功能:
1. 创建一个新的WinForms项目。
2. 安装OxyPlot和OxyPlot.WinForms NuGet包。
3. 添加一个`PlotView`控件到你的表单中。
4. 编写代码来生成和更新瀑布图。
### 第一步:安装NuGet包
打开NuGet包管理器控制台并运行以下命令:
```shell
Install-Package OxyPlot.Core
Install-Package OxyPlot.WindowsForms
```
### 第二步:设计表单
在你的表单上添加一个`PlotView`控件。你可以通过拖放工具从工具箱中找到它,或者手动在代码中添加。
### 第三步:编写代码
下面是一个完整的示例代码,展示如何实现每秒更新5000个数据点的瀑布图:
```csharp
using System;
using System.Collections.Generic;
using System.Linq;
using System.Windows.Forms;
using OxyPlot;
using OxyPlot.Series;
using OxyPlot.Axes;
using OxyPlot.WindowsForms;
namespace WaterfallChartExample
{
public partial class Form1 : Form
{
private PlotView plotView;
private LineSeries lineSeries;
private Random random = new Random();
private List<double> dataPoints = new List<double>();
private int counter = 0;
public Form1()
{
InitializeComponent();
InitializePlot();
}
private void InitializePlot()
{
plotView = new PlotView
{
Dock = DockStyle.Fill,
Location = new System.Drawing.Point(0, 0),
Name = "plotView",
Size = new System.Drawing.Size(800, 600),
TabIndex = 0,
Text = "plotView"
};
this.Controls.Add(plotView);
var model = new PlotModel { Title = "Waterfall Chart Example" };
lineSeries = new LineSeries { MarkerType = MarkerType.None };
model.Series.Add(lineSeries);
plotView.Model = model;
// Set up the timer to update the chart every second
var timer = new System.Windows.Forms.Timer();
timer.Interval = 1000; // 1 second
timer.Tick += (sender, e) => UpdateChart();
timer.Start();
}
private void UpdateChart()
{
for (int i = 0; i < 5000; i++)
{
double newValue = random.NextDouble() * 100; // Generate a random value between 0 and 100
dataPoints.Add(newValue);
lineSeries.Points.Add(new DataPoint(counter++, newValue));
}
// Keep only the last 5000 points in the series to avoid memory issues
if (lineSeries.Points.Count > 5000)
{
lineSeries.Points.RemoveAt(0);
}
plotView.InvalidatePlot(true); // Redraw the plot
}
}
}
```
### 解释代码
1. **初始化PlotView**:在`InitializePlot`方法中,我们创建了一个`PlotView`控件并将其添加到表单中。我们还创建了一个`PlotModel`和一个`LineSeries`,并将它们关联起来。
2. **定时器**:使用`System.Windows.Forms.Timer`来每秒调用一次`UpdateChart`方法。
3. **更新图表**:在`UpdateChart`方法中,我们生成5000个随机数据点,并将它们添加到`LineSeries`中。如果数据点的数量超过5000,我们移除最早的数据点以保持性能。最后,我们调用`InvalidatePlot`方法重新绘制图表。
通过这种方式,你可以实现一个每秒更新5000个数据点的瀑布图。
阅读全文
相关推荐
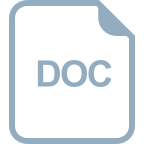
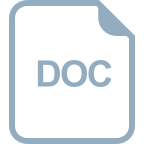
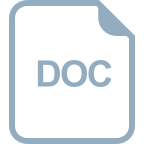




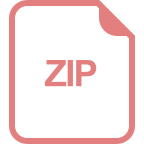
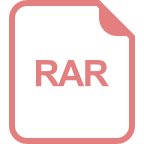




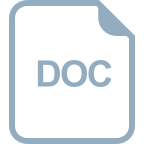
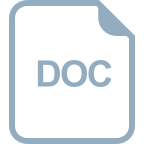
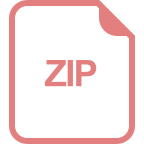