c++要怎么返回结构体
时间: 2024-08-15 10:06:04 浏览: 49
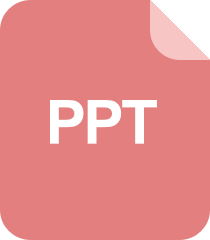
进入C++的基础语法-C++结构体和公用体
在C++中,返回结构体的基本方式是在函数定义时声明该函数可以返回所需的数据类型——在这种情况下,就是结构体。
假设我们有一个名为`MyStruct`的结构体:
```cpp
struct MyStruct {
int id;
std::string name;
};
```
我们可以创建一个函数来返回这个结构体:
```cpp
MyStruct createMyStruct(int id, const std::string& name) {
MyStruct structData;
structData.id = id;
structData.name = name;
return structData;
}
```
为了调用此函数并返回结果到C#或其他语言中,我们需要编写一个桥接函数或使用某种机制来进行类型转换。如果直接调用,可能需要利用Windows API的`GetProcAddress`与`LoadLibrary`来实现动态链接,或者使用C++/CLI(如果在Visual Studio环境下)等技术,使得C++函数可以直接被.NET框架调用而无需额外的类型转换。
### 使用C++/CLI的例子
如果在Visual Studio环境下使用C++/CLI,可以在C++函数前面添加`__declspec(dllexport)`以允许从.NET程序访问它:
```cpp
#include <iostream>
using namespace System;
// Define the structure using PInvoke convention
[StructLayout(LayoutKind.Sequential)]
public ref class MyStruct {
public:
int Id;
String^ Name;
};
[STAThread]
int main(array<System::String ^> ^args)
{
// Call our function and convert the result to a format understood by .NET.
MyStruct^ myStruct = gcnew MyStruct();
myStruct->Id = 1;
myStruct->Name = "Example";
// Now you can pass this struct back to your .NET application as needed.
return 0;
}
__declspec(dllexport) MyStruct createMyStruct(int id, String^ name)
{
MyStruct structData;
structData.Id = id;
structData.Name = gcnew String(name);
return structData;
}
```
在这个例子中,`createMyStruct`函数返回一个`MyStruct`实例,可以直接由.NET应用使用。需要注意的是,在实际生产环境中,通常会依赖于特定的库或中间层来简化这种跨语言通信的过程。
---
## 相关问题 - :
1. 如何在C++中安全地管理结构体数组的内存?
2. 当在C++中向C#传递复杂数据类型如自定义结构体时,应考虑哪些关键因素?
3. 在不使用C++/CLI的情况下,如何在C#中调用C++ DLL中包含结构体返回的函数?
阅读全文
相关推荐
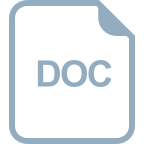
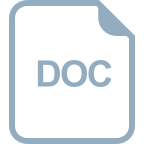






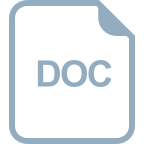







