用c语言编程实现输入某年某月某天,求这个日期在该年的第几天?
时间: 2024-10-08 11:13:45 浏览: 7
在C语言中,你可以通过编写一个函数来实现这个功能,通常会涉及到对闰年的判断和月份天数的计算。这里提供一个简单的示例:
```c
#include <stdio.h>
// Function to calculate the day of the year
int daysInMonth(int month, int year) {
switch(month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
return 31;
case 4:
case 6:
case 9:
case 11:
return 30;
// February needs to check for leap year
case 2:
if (year % 4 == 0 && (year % 100 != 0 || year % 400 == 0)) {
return 29; // Leap year
} else {
return 28;
}
default:
return -1; // Invalid month
}
}
// Main function
int main() {
int year, month, day;
printf("Enter a date in format (YYYY MM DD): ");
scanf("%d %d %d", &year, &month, &day);
// Check input validity
if(month > 12 || day > daysInMonth(month, year)) {
printf("Invalid date.\n");
return 1;
}
int total_days = 0;
for (int i = 1; i < month; ++i) {
total_days += daysInMonth(i, year);
}
total_days += day;
printf("The given date is the %dth day of the year.\n", total_days);
return 0;
}
```
在这个程序中,`daysInMonth` 函数负责计算给定月份的天数,包括闰年的情况。主函数`main`则接收用户输入,验证日期的有效性,并计算出该日期在那一年中的位置。
相关推荐
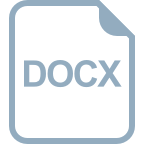
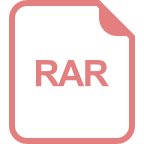














