import pandas as pd import numpy as np import matplotlib.pyplot as plt from mpl_toolkits.mplot3d import Axes3D features = df[["Bridge length (m)","Pier type","Foundation type","Hole","Span (m)", "Bearing type","Plane linear"]] target = data['Vulnerability grade'] # 生成测试数据网格 x = np.linspace(features['feature1'].min(), features['feature1'].max(), 100) y = np.linspace(features['feature2'].min(), features['feature2'].max(), 100) z = np.linspace(features['feature3'].min(), features['feature3'].max(), 100) X_test = np.meshgrid(x, y, z) X_test = np.vstack((X_test[0].flatten(), X_test[1].flatten(), X_test[2].flatten())).T # 绘制预测结果的空间曲面 fig = plt.figure() ax = fig.add_subplot(111, projection='3d') ax.scatter(features['feature1'], features['feature2'], features['feature3'], c=target, cmap='coolwarm', label='Training Data') ax.scatter(X_test[:, 0], X_test[:, 1], X_test[:, 2], c=test_predicted, cmap='coolwarm', label='Predicted Data') ax.set_xlabel('Feature 1') ax.set_ylabel('Feature 2') ax.set_zlabel('Feature 3') ax.legend() plt.show()
时间: 2024-04-23 13:27:56 浏览: 17
在您提供的代码中,有一些问题需要修正。首先,您将特征列存储在`features`变量中,但在生成测试数据网格时,您使用了`features['feature1']`等特征名,这可能是导致KeyError的原因。另外,您没有给出训练模型和进行预测的代码。以下是修正后的示例代码:
```python
import pandas as pd
import numpy as np
import matplotlib.pyplot as plt
from sklearn.ensemble import RandomForestRegressor
from mpl_toolkits.mplot3d import Axes3D
# 读取数据
data = pd.read_excel('your_data.xlsx') # 替换为您的Excel文件路径
# 提取特征和目标变量
features = data[["Bridge length (m)", "Pier type", "Foundation type", "Hole", "Span (m)", "Bearing type", "Plane linear"]]
target = data['Vulnerability grade']
# 训练随机森林回归模型
rf = RandomForestRegressor(n_estimators=100, random_state=0)
rf.fit(features, target)
# 生成测试数据网格
x = np.linspace(features['Bridge length (m)'].min(), features['Bridge length (m)'].max(), 100)
y = np.linspace(features['Pier type'].min(), features['Pier type'].max(), 100)
z = np.linspace(features['Foundation type'].min(), features['Foundation type'].max(), 100)
X_test = np.meshgrid(x, y, z)
X_test = np.vstack((X_test[0].flatten(), X_test[1].flatten(), X_test[2].flatten())).T
# 使用随机森林模型进行预测
test_predicted = rf.predict(X_test)
# 绘制预测结果的空间曲面
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.scatter(features['Bridge length (m)'], features['Pier type'], features['Foundation type'], c=target, cmap='coolwarm', label='Training Data')
ax.scatter(X_test[:, 0], X_test[:, 1], X_test[:, 2], c=test_predicted, cmap='coolwarm', label='Predicted Data')
ax.set_xlabel('Bridge length (m)')
ax.set_ylabel('Pier type')
ax.set_zlabel('Foundation type')
ax.legend()
plt.show()
```
请注意,根据您的数据和需求,您可能需要调整代码中的特征列名、文件路径以及其他绘图参数。确保特征列和目标变量与您的数据一致,并且选择合适的特征列用于绘制空间曲面。
相关推荐
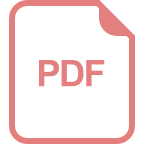
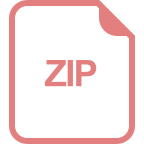
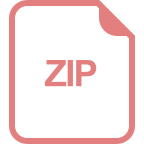














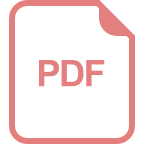