编写一个函数 read_file_lines(file_path),使用 open() 函数逐行读取一个已存在example.txt文件内容,并将每一行打印到控制台,添加行号。
时间: 2024-11-30 13:19:36 浏览: 36
```python
def read_file_lines(file_path):
try:
with open(file_path, 'r') as file:
for line_number, line in enumerate(file, start=1): # 使用enumerate从1开始计数行号
print(f"Line {line_number}: {line.strip()}") # .strip()用于移除行尾的换行符
except FileNotFoundError:
print(f"File '{file_path}' not found.")
except Exception as e:
print(f"An error occurred while reading the file: {e}")
# 调用函数并传入示例文件路径
read_file_lines('example.txt')
```
这个函数首先尝试打开指定的文件,然后使用`enumerate()`遍历文件内容,同时记录每行的行号。如果文件不存在,会捕获`FileNotFoundError`;其他未知错误则通过`Exception`处理。每行的内容会被打印到控制台,包括自动生成的行号。
相关问题
用python定义一个读取txt文件的函数,用来读取txt文件内的数据
在Python中,你可以定义一个简单的函数,使用内置的`open()`函数打开txt文件并逐行读取内容。这里是一个基础示例:
```python
def read_txt_file(file_path):
"""
用于读取txt文件的函数
参数:
file_path (str): 需要读取的txt文件路径
返回:
list: txt文件中每行的内容,作为一个列表
"""
try:
with open(file_path, 'r', encoding='utf-8') as file:
lines = file.readlines()
# 如果不需要去除换行符,可以直接返回lines
# 如果需要处理每一行的数据,可以遍历并操作list,例如清洗、解析等
data = [line.strip() for line in lines] # 去除换行符
return data
except FileNotFoundError:
print(f"文件 {file_path} 未找到")
except Exception as e:
print(f"读取文件时发生错误:{e}")
# 使用函数
data = read_txt_file('example.txt')
python 使用yield函数读取txt文件
在Python中,可以使用yield函数来逐行读取txt文件。yield函数是一个生成器函数,它可以暂停执行并返回一个值,然后再次从暂停的位置继续执行。
下面是一个示例代码,演示如何使用yield函数读取txt文件:
```python
def read_file(file_path):
with open(file_path, 'r') as file:
for line in file:
yield line.strip()
file_path = 'example.txt'
lines = read_file(file_path)
for line in lines:
print(line)
```
在上面的代码中,read_file函数是一个生成器函数,它打开指定的txt文件,并使用for循环逐行读取文件内容。每次读取一行后,使用yield语句将该行返回。然后,在主程序中,我们可以通过迭代lines来获取每一行的内容,并进行相应的处理。
阅读全文
相关推荐
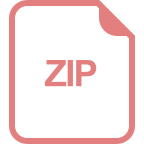
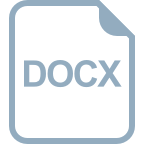
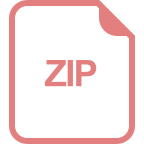
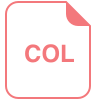
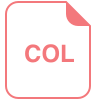
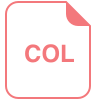
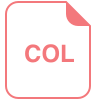
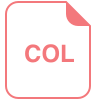








