VS2019 C++ 通过 filesystem 函数对.txt文件删除行数据怎么操作,用代码说明
时间: 2024-10-22 17:15:26 浏览: 38
在Visual Studio 2019的C++中,如果你想使用`filesystem`库来读取`.txt`文件并删除指定行的数据,首先你需要包含头文件`#include <filesystem>`(如果你使用的是C++17及以上版本),然后可以按照以下步骤操作:
```cpp
#include <iostream>
#include <fstream>
#include <string>
#include <vector>
#include <filesystem>
namespace fs = std::filesystem;
// 读取文件内容到字符串向量中
std::vector<std::string> read_file_lines(const fs::path& filePath) {
std::ifstream file(filePath);
std::vector<std::string> lines;
if (file.is_open()) {
std::string line;
while (std::getline(file, line)) {
lines.push_back(line);
}
file.close();
} else {
std::cerr << "Failed to open the file." << std::endl;
}
return lines;
}
// 删除指定行
void delete_line(std::vector<std::string>& lines, size_t index) {
if (index < lines.size()) {
lines.erase(lines.begin() + index);
} else {
std::cout << "Line index out of range." << std::endl;
}
}
// 写回文件
void write_file(const fs::path& filePath, const std::vector<std::string>& newLines) {
std::ofstream file(filePath);
if (file.is_open()) {
for (const auto& line : newLines) {
file << line << "\n";
}
file.close();
} else {
std::cerr << "Failed to open the file for writing." << std::endl;
}
}
int main() {
try {
// 例子:假设我们想要从文件"example.txt"中删除第5行
fs::path filePath = "example.txt";
// 读取原始文件
std::vector<std::string> originalLines = read_file_lines(filePath);
// 删除指定行
delete_line(originalLines, 4); // 行索引从0开始
// 保存修改后的文件
write_file(filePath, originalLines);
std::cout << "Line deletion successful.\n";
} catch (const fs::exception& e) {
std::cerr << "Error with file operations: " << e.what() << std::endl;
}
return 0;
}
```
这个程序首先读取文本文件的所有行,然后删除指定行,最后将剩下的行写回到原文件。注意,这里删除的是基于零的索引,即第一行是0。
阅读全文
相关推荐
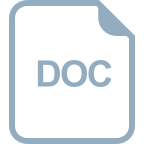
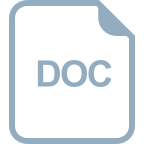
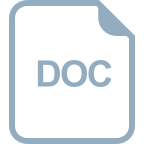





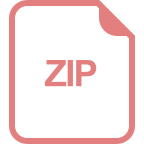







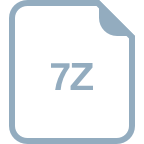

