用C++作出如下要求(1)初始化顺序表。 (2)在顺序表的第 i 个位置插入元素。 (3)删除顺序表的第 i 个元素。 (4)输出顺序表。 (5)判断顺序表是否为空。 (6)判断顺序表是否为满。 (7)求顺序表第 i 个元素的值。 (8)查找值为 x 的元素。
时间: 2024-09-29 07:13:03 浏览: 24
在C++中,我们可以使用`std::vector`作为动态数组来实现顺序表的功能,因为它提供了方便的插入、删除和访问操作。以下是针对你所提要求的一些基本实现:
1. 初始化顺序表:
```cpp
std::vector<int> sequence; // 创建空的顺序表
sequence.reserve(size); // 如果知道预估大小,可以预先分配空间
```
2. 插入元素到第 i 个位置:
```cpp
void insert(int value, int index) {
if (index < 0 || index > sequence.size()) {
std::cout << "Invalid index!";
} else {
sequence.insert(sequence.begin() + index, value);
}
}
```
3. 删除第 i 个元素:
```cpp
void remove(int index) {
if (index < 0 || index >= sequence.size()) {
std::cout << "Invalid index!";
} else {
sequence.erase(sequence.begin() + index);
}
}
```
4. 输出顺序表:
```cpp
void print_sequence() {
for (const auto &element : sequence) {
std::cout << element << " ";
}
std::cout << "\n";
}
```
5. 判断顺序表是否为空:
```cpp
bool is_empty() const {
return sequence.empty();
}
```
6. 判断顺序表是否为满(假设我们有一个固定大小的顺序表):
```cpp
bool is_full(const size_t capacity) const {
return sequence.size() == capacity;
}
```
7. 获取第 i 个元素的值:
```cpp
int get_value(int index) const {
if (index < 0 || index >= sequence.size()) {
throw std::out_of_range("Index out of range");
}
return sequence[index];
}
```
8. 查找值为 x 的元素:
```cpp
int find_value(int target) const {
for (size_t i = 0; i < sequence.size(); ++i) {
if (sequence[i] == target) {
return i;
}
}
return -1; // 如果未找到目标,返回 -1 或者抛异常
}
```
阅读全文
相关推荐
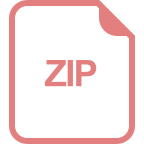
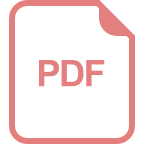
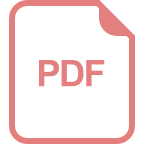















