opencvc++人脸识别
时间: 2024-01-13 11:19:54 浏览: 42
以下是使用OpenCV和C++实现人脸识别的示例代码:
```cpp
#include <opencv2/opencv.hpp>
#include <opencv2/highgui.hpp>
#include <opencv2/face.hpp>
using namespace cv;
using namespace cv::face;
int main()
{
// 加载人脸识别模型
Ptr<LBPHFaceRecognizer> model = LBPHFaceRecognizer::create();
model->read("path_to_model.xml");
// 加载人脸检测器
CascadeClassifier faceCascade;
faceCascade.load("path_to_cascade.xml");
// 打开摄像头
VideoCapture capture(0);
if (!capture.isOpened())
{
std::cout << "无法打开摄像头" << std::endl;
return -1;
}
while (true)
{
Mat frame;
capture >> frame;
// 转换为灰度图像
Mat gray;
cvtColor(frame, gray, COLOR_BGR2GRAY);
// 检测人脸
std::vector<Rect> faces;
faceCascade.detectMultiScale(gray, faces, 1.1, 3, 0, Size(100, 100));
for (const auto& face : faces)
{
// 绘制人脸矩形框
rectangle(frame, face, Scalar(0, 255, 0), 2);
// 人脸识别
Mat faceROI = gray(face);
int label;
double confidence;
model->predict(faceROI, label, confidence);
// 显示识别结果
std::string result = "Label: " + std::to_string(label) + ", Confidence: " + std::to_string(confidence);
putText(frame, result, Point(face.x, face.y - 10), FONT_HERSHEY_SIMPLEX, 0.9, Scalar(0, 255, 0), 2);
}
// 显示图像
imshow("Face Recognition", frame);
// 按下ESC键退出
if (waitKey(1) == 27)
break;
}
// 释放摄像头和窗口
capture.release();
destroyAllWindows();
return 0;
}
```
请注意,上述代码中的`path_to_model.xml`和`path_to_cascade.xml`需要替换为实际的模型和级联分类器文件的路径。
相关推荐
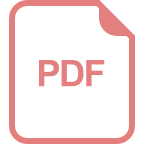
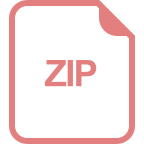
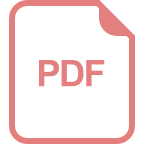














