C# TCPlistener 接收多个连接
时间: 2024-10-05 19:04:31 浏览: 44
在C#中,`TcpListener`可以同时处理多个连接,因为它的`AcceptTcpClient()`方法是异步的,这意味着它不会阻塞当前线程,而是返回一个`Task`或`TcpClient`实例,你可以通过回调函数或事件处理的方式来处理新的连接请求。这样,主线程可以继续监听新的连接,而不需要等待每一个连接完成。
以下是一个简化版的示例,展示了如何在一个单独的线程中接收多个连接:
```csharp
using System;
using System.Collections.Concurrent;
using System.Net;
using System.Net.Sockets;
using System.Threading.Tasks;
public class MultiConnectionHandler
{
private TcpListener _listener;
private BlockingCollection<TcpClient> _clients;
public MultiConnectionHandler(int port)
{
_listener = new TcpListener(IPAddress.Any, port);
_clients = new BlockingCollection<TcpClient>();
}
public async Task StartListening()
{
_listener.Start();
Console.WriteLine($"Listening on {(_listener.LocalEndpoint)}");
while (true)
{
// 异步接受新的连接并添加到队列
await AcceptNewConnectionAsync();
}
}
private async Task AcceptNewConnectionAsync()
{
try
{
TcpClient client = await _listener.AcceptTcpClientAsync();
_clients.Add(client);
Console.WriteLine($"Accepted connection from {client.Client.RemoteEndPoint}");
}
catch (Exception ex)
{
Console.WriteLine($"Error accepting connection: {ex.Message}");
}
}
public async Task HandleConnections()
{
while (_clients.TryTake(out TcpClient client))
{
// 在这里处理每个连接,例如读取数据、发送响应等
using (NetworkStream stream = client.GetStream())
{
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = await stream.ReadAsync(buffer, 0, buffer.Length)) > 0)
{
// 接收客户端的数据
// ...
// 发送响应
// ...
}
}
// 关闭连接后从集合移除
client.Close();
}
}
public void StopListening()
{
_listener.Stop();
}
}
// 主程序入口
public class Program
{
public static void Main(string[] args)
{
var handler = new MultiConnectionHandler(8080);
Task.Run(() => handler.StartListening());
handler.HandleConnections().Wait();
}
}
```
在这个示例中,`StartListening`任务负责持续监听新连接,`HandleConnections`任务则负责处理队列中的每一个连接。当需要停止监听时,调用`StopListening`方法即可。
阅读全文
相关推荐
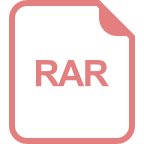
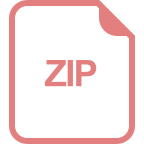
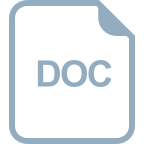
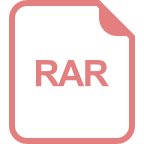

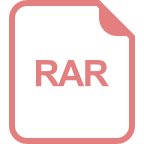
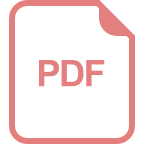
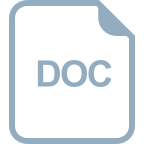
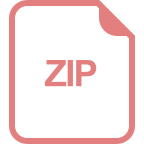
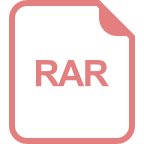
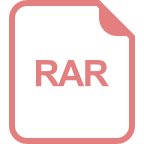
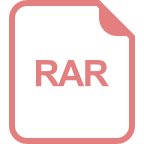
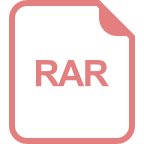
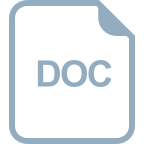
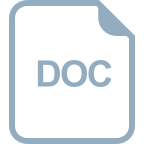
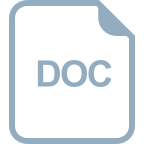
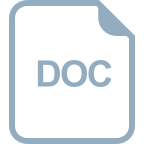
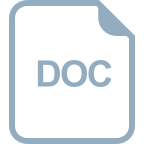
