python粒子特效
时间: 2024-01-25 16:13:21 浏览: 43
以下是一个使用Python实现的简单粒子特效的例子:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置窗口大小和标题
width, height = 800, 600
screen = pygame.display.set_mode((width, height))
pygame.display.set_caption("Particle Effect")
# 定义粒子类
class Particle:
def __init__(self, x, y):
self.x = x
self.y = y
self.size = random.randint(1, 5)
self.color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
self.speed_x = random.uniform(-1, 1)
self.speed_y = random.uniform(-1, 1)
self.lifetime = random.randint(30, 60)
def update(self):
self.x += self.speed_x
self.y += self.speed_y
self.lifetime -= 1
def draw(self):
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), self.size)
# 创建粒子列表
particles = []
# 游戏主循环
running = True
while running:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# 更新粒子
for particle in particles:
particle.update()
if particle.lifetime <= 0:
particles.remove(particle)
# 生成新粒子
if random.random() < 0.1:
particle = Particle(width/2, height/2)
particles.append(particle)
# 绘制粒子
screen.fill((0, 0, 0))
for particle in particles:
particle.draw()
# 更新屏幕
pygame.display.flip()
# 退出游戏
pygame.quit()
```
这个例子使用了pygame库来创建一个窗口,并在窗口中实现了一个简单的粒子特效。粒子通过随机生成的速度和颜色,在屏幕上移动并渐渐消失。你可以根据自己的需求调整粒子的数量、速度范围、颜色范围等参数来实现不同的效果。
相关推荐
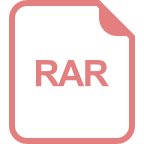
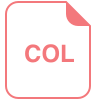
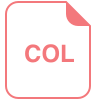
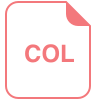
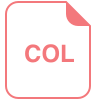
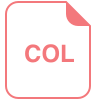









