数据结构c语言程序:一个线性表L中的数据元素按升序排列,编写一个算法,实现在线性表中插入一个数据元素item,使得线性表仍然保持升序排列。
时间: 2024-09-15 20:08:09 浏览: 41
在C语言中,如果你需要在已排序的线性表(通常是数组或链表)中插入一个新元素并保持升序,你可以选择以下几种策略:
1. **数组**:
如果是动态数组,你需要遍历整个数组找到插入位置。从数组末尾开始比较 `item` 和现有元素,如果 `item` 小于当前元素,就向后移动一位直到找到合适的位置,然后将所有大于 `item` 的元素依次后移一位。
```c
void insert_sorted(int arr[], int n, int item) {
for (int i = n - 1; i >= 0 && arr[i] > item; i--) {
arr[i + 1] = arr[i];
}
arr[i + 1] = item;
}
```
2. **链表**:
对于单链表,可以使用类似数组的方法,但在遍历时只能通过指针前移。找到适当位置后插入新节点,并调整相邻节点的指针。
```c
typedef struct Node {
int data;
struct Node *next;
} Node;
void insert_sorted_list(Node **head, int item) {
if (*head == NULL || item <= (*head)->data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = item;
newNode->next = *head;
*head = newNode;
} else {
Node* current = *head;
while (current->next != NULL && current->next->data < item) {
current = current->next;
}
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = item;
newNode->next = current->next;
current->next = newNode;
}
}
```
阅读全文
相关推荐
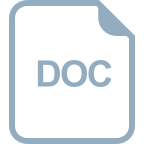
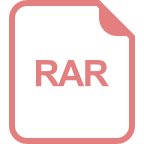
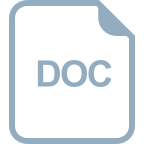
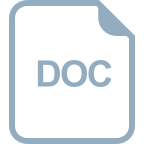
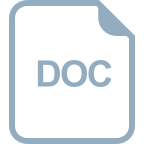
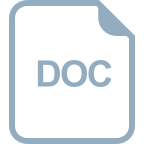
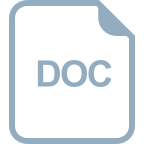
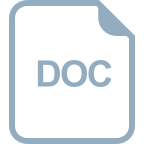
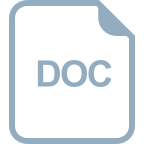
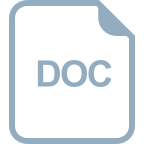
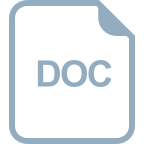
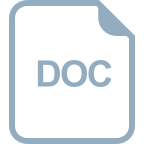
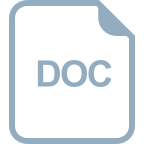
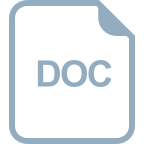
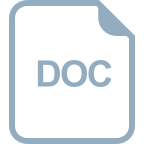
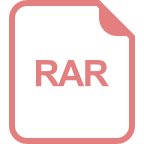
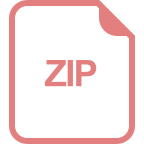