C语言线性表的应用算法:构造两个按指数递增的有序链表,实现两个一元多项式相加
时间: 2024-06-13 14:07:01 浏览: 12
以下是C语言线性表的应用算法:构造两个按指数递增的有序链表,实现两个一元多项式相加的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct node {
int coef; // 系数
int expn; // 指数
struct node *next; // 指向下一个节点的指针
} Node, *LinkList;
// 创建一个新节点
Node *newNode(int coef, int expn) {
Node *node = (Node *)malloc(sizeof(Node));
node->coef = coef;
node->expn = expn;
node->next = NULL;
return node;
}
// 创建一个新链表
LinkList newLinkList() {
LinkList list = (LinkList)malloc(sizeof(Node));
list->next = NULL;
return list;
}
// 向链表中插入一个节点
void insert(LinkList list, Node *node) {
Node *p = list;
while (p->next && p->next->expn < node->expn) {
p = p->next;
}
if (p->next && p->next->expn == node->expn) {
p->next->coef += node->coef;
if (p->next->coef == 0) {
Node *temp = p->next;
p->next = temp->next;
free(temp);
}
} else {
node->next = p->next;
p->next = node;
}
}
// 从标准输入中读取一个多项式
LinkList readPoly() {
LinkList list = newLinkList();
int n;
scanf("%d", &n);
for (int i = 0; i < n; i++) {
int coef, expn;
scanf("%d %d", &coef, &expn);
insert(list, newNode(coef, expn));
}
return list;
}
// 打印一个多项式
void printPoly(LinkList list) {
Node *p = list->next;
while (p) {
printf("%d %d ", p->coef, p->expn);
p = p->next;
}
printf("\n");
}
// 多项式相加
LinkList addPoly(LinkList list1, LinkList list2) {
LinkList list = newLinkList();
Node *p1 = list1->next, *p2 = list2->next;
while (p1 && p2) {
if (p1->expn < p2->expn) {
insert(list, newNode(p1->coef, p1->expn));
p1 = p1->next;
} else if (p1->expn > p2->expn) {
insert(list, newNode(p2->coef, p2->expn));
p2 = p2->next;
} else {
insert(list, newNode(p1->coef + p2->coef, p1->expn));
p1 = p1->next;
p2 = p2->next;
}
}
while (p1) {
insert(list, newNode(p1->coef, p1->expn));
p1 = p1->next;
}
while (p2) {
insert(list, newNode(p2->coef, p2->expn));
p2 = p2->next;
}
return list;
}
int main() {
LinkList list1 = readPoly();
LinkList list2 = readPoly();
LinkList list = addPoly(list1, list2);
printPoly(list);
return 0;
}
```
相关推荐
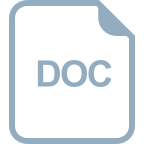
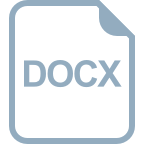














