一元多项式的相加:选用适当的线性表结构存储一元多项式,并实现两个多项式相加,并将结果按照数学公式格式输出。多项式的数据应由键盘输入。
时间: 2024-02-19 13:02:55 浏览: 270
好的,我可以帮你解决这个问题。对于一元多项式的存储,我们可以使用线性表中的顺序存储结构或链式存储结构。这里我以链式存储结构为例。
我们可以定义一个结构体来表示一元多项式的每一项,包括系数和指数:
```
typedef struct PolyNode
{
float coef; // 系数
int expn; // 指数
struct PolyNode *next;
} PolyNode, *Polynomial;
```
其中,`coef`表示系数,`expn`表示指数,`next`表示下一项元素的指针。
接下来,我们可以编写一个函数来创建多项式:
```
Polynomial CreatePoly()
{
Polynomial head, tail, node;
int coef, expn;
head = (Polynomial)malloc(sizeof(PolyNode));
head->next = NULL;
tail = head;
printf("请输入多项式系数和指数(以0 0结束):\n");
while (scanf("%d %d", &coef, &expn) == 2 && coef != 0)
{
node = (Polynomial)malloc(sizeof(PolyNode));
node->coef = coef;
node->expn = expn;
tail->next = node;
tail = node;
}
tail->next = NULL;
return head;
}
```
该函数会从键盘输入多项式的系数和指数,以0 0结束,并将其保存在一个链表中。
然后,我们可以编写一个函数来实现多项式相加:
```
Polynomial AddPoly(Polynomial P1, Polynomial P2)
{
Polynomial head, tail, node;
head = (Polynomial)malloc(sizeof(PolyNode));
head->next = NULL;
tail = head;
while (P1 && P2)
{
if (P1->expn == P2->expn)
{
float sum = P1->coef + P2->coef;
if (sum != 0)
{
node = (Polynomial)malloc(sizeof(PolyNode));
node->coef = sum;
node->expn = P1->expn;
tail->next = node;
tail = node;
}
P1 = P1->next;
P2 = P2->next;
}
else if (P1->expn > P2->expn)
{
node = (Polynomial)malloc(sizeof(PolyNode));
node->coef = P1->coef;
node->expn = P1->expn;
tail->next = node;
tail = node;
P1 = P1->next;
}
else
{
node = (Polynomial)malloc(sizeof(PolyNode));
node->coef = P2->coef;
node->expn = P2->expn;
tail->next = node;
tail = node;
P2 = P2->next;
}
}
while (P1)
{
node = (Polynomial)malloc(sizeof(PolyNode));
node->coef = P1->coef;
node->expn = P1->expn;
tail->next = node;
tail = node;
P1 = P1->next;
}
while (P2)
{
node = (Polynomial)malloc(sizeof(PolyNode));
node->coef = P2->coef;
node->expn = P2->expn;
tail->next = node;
tail = node;
P2 = P2->next;
}
tail->next = NULL;
return head;
}
```
该函数接受两个多项式作为参数,返回它们相加的结果。在这个函数中,我们遍历两个多项式,逐项进行比较,将结果保存在一个新的链表中。
最后,我们可以编写一个函数来按照数学公式格式输出多项式:
```
void PrintPoly(Polynomial P)
{
if (!P)
{
printf("0\n");
return;
}
int flag = 0;
while (P)
{
if (P->coef > 0 && flag != 0)
{
printf("+");
}
if (P->expn == 0)
{
printf("%.2f", P->coef);
}
else if (P->expn == 1)
{
printf("%.2fx", P->coef);
}
else
{
printf("%.2fx^%d", P->coef, P->expn);
}
P = P->next;
flag++;
}
printf("\n");
}
```
该函数遍历多项式,并按照数学公式格式输出多项式。
最后,我们可以在主函数中调用上述函数,实现多项式相加并输出结果,代码如下:
```
int main()
{
Polynomial P1, P2, P3;
printf("请输入第一个多项式:\n");
P1 = CreatePoly();
printf("请输入第二个多项式:\n");
P2 = CreatePoly();
P3 = AddPoly(P1, P2);
printf("相加的结果为:\n");
PrintPoly(P3);
return 0;
}
```
希望能对你有所帮助!
阅读全文
相关推荐
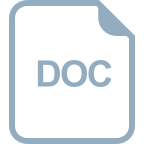
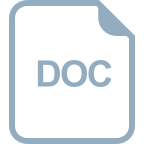
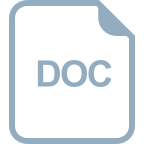
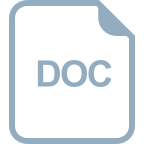
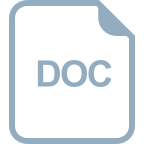
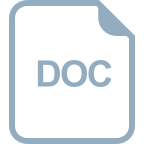
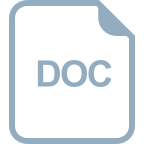
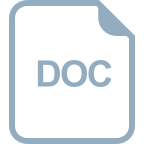
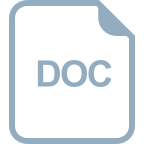
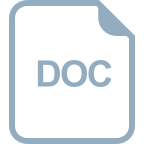
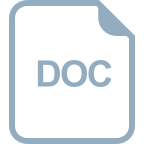
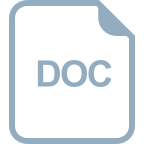
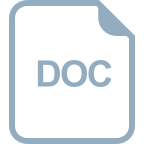
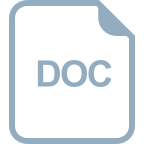
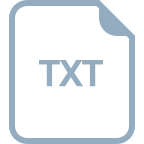